List elements digit difference sort
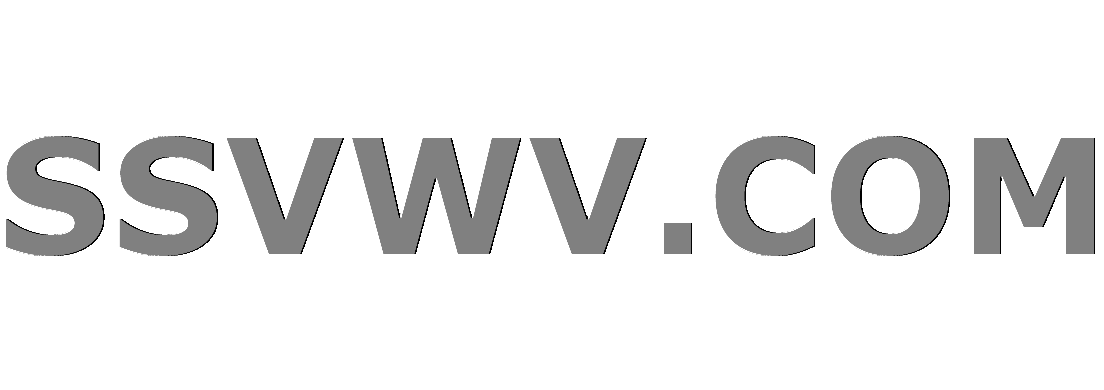
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ margin-bottom:0;
}
$begingroup$
I am doing a code challenge of codesignal.com which ask for following things:
Given an array of integers, sort its elements by the difference of their largest and smallest digits. In the case of a tie, that with the larger index in the array should come first.
Example
For a = [152, 23, 7, 887, 243], the output should be
digitDifferenceSort(a) = [7, 887, 23, 243, 152].
Here are the differences of all the numbers:
152: difference = 5 - 1 = 4;
23: difference = 3 - 2 = 1;
7: difference = 7 - 7 = 0;
887: difference = 8 - 7 = 1;
243: difference = 4 - 2 = 2.
23 and 887 have the same difference, but 887 goes after 23 in a, so in the sorted array it comes first.
I wrote following code using python3 and it passes all normal tests but it cannot pass execution time tests. How can I improve my code to decrease it's execution time for list with considerable amount of elements?
def digitDifferenceSort(a):
diff =
for i in a:
i = list(str(i))
diff.append(i)
for i in range(len(diff)):
for j in range(i+1, len(diff)):
if int(max(diff[i])) - int(min(diff[i])) > int(max(diff[j])) - int(min(diff[j])):
diff[j], diff[i] = diff[i], diff[j]
elif int(max(diff[i])) - int(min(diff[i])) == int(max(diff[j])) - int(min(diff[j])):
diff[i], diff[j] = diff[j], diff[i]
new_list =
for i in diff:
b = ''
for j in i:
b = b + j
new_list.append(int(b))
return new_list
python python-3.x time-limit-exceeded
$endgroup$
add a comment |
$begingroup$
I am doing a code challenge of codesignal.com which ask for following things:
Given an array of integers, sort its elements by the difference of their largest and smallest digits. In the case of a tie, that with the larger index in the array should come first.
Example
For a = [152, 23, 7, 887, 243], the output should be
digitDifferenceSort(a) = [7, 887, 23, 243, 152].
Here are the differences of all the numbers:
152: difference = 5 - 1 = 4;
23: difference = 3 - 2 = 1;
7: difference = 7 - 7 = 0;
887: difference = 8 - 7 = 1;
243: difference = 4 - 2 = 2.
23 and 887 have the same difference, but 887 goes after 23 in a, so in the sorted array it comes first.
I wrote following code using python3 and it passes all normal tests but it cannot pass execution time tests. How can I improve my code to decrease it's execution time for list with considerable amount of elements?
def digitDifferenceSort(a):
diff =
for i in a:
i = list(str(i))
diff.append(i)
for i in range(len(diff)):
for j in range(i+1, len(diff)):
if int(max(diff[i])) - int(min(diff[i])) > int(max(diff[j])) - int(min(diff[j])):
diff[j], diff[i] = diff[i], diff[j]
elif int(max(diff[i])) - int(min(diff[i])) == int(max(diff[j])) - int(min(diff[j])):
diff[i], diff[j] = diff[j], diff[i]
new_list =
for i in diff:
b = ''
for j in i:
b = b + j
new_list.append(int(b))
return new_list
python python-3.x time-limit-exceeded
$endgroup$
add a comment |
$begingroup$
I am doing a code challenge of codesignal.com which ask for following things:
Given an array of integers, sort its elements by the difference of their largest and smallest digits. In the case of a tie, that with the larger index in the array should come first.
Example
For a = [152, 23, 7, 887, 243], the output should be
digitDifferenceSort(a) = [7, 887, 23, 243, 152].
Here are the differences of all the numbers:
152: difference = 5 - 1 = 4;
23: difference = 3 - 2 = 1;
7: difference = 7 - 7 = 0;
887: difference = 8 - 7 = 1;
243: difference = 4 - 2 = 2.
23 and 887 have the same difference, but 887 goes after 23 in a, so in the sorted array it comes first.
I wrote following code using python3 and it passes all normal tests but it cannot pass execution time tests. How can I improve my code to decrease it's execution time for list with considerable amount of elements?
def digitDifferenceSort(a):
diff =
for i in a:
i = list(str(i))
diff.append(i)
for i in range(len(diff)):
for j in range(i+1, len(diff)):
if int(max(diff[i])) - int(min(diff[i])) > int(max(diff[j])) - int(min(diff[j])):
diff[j], diff[i] = diff[i], diff[j]
elif int(max(diff[i])) - int(min(diff[i])) == int(max(diff[j])) - int(min(diff[j])):
diff[i], diff[j] = diff[j], diff[i]
new_list =
for i in diff:
b = ''
for j in i:
b = b + j
new_list.append(int(b))
return new_list
python python-3.x time-limit-exceeded
$endgroup$
I am doing a code challenge of codesignal.com which ask for following things:
Given an array of integers, sort its elements by the difference of their largest and smallest digits. In the case of a tie, that with the larger index in the array should come first.
Example
For a = [152, 23, 7, 887, 243], the output should be
digitDifferenceSort(a) = [7, 887, 23, 243, 152].
Here are the differences of all the numbers:
152: difference = 5 - 1 = 4;
23: difference = 3 - 2 = 1;
7: difference = 7 - 7 = 0;
887: difference = 8 - 7 = 1;
243: difference = 4 - 2 = 2.
23 and 887 have the same difference, but 887 goes after 23 in a, so in the sorted array it comes first.
I wrote following code using python3 and it passes all normal tests but it cannot pass execution time tests. How can I improve my code to decrease it's execution time for list with considerable amount of elements?
def digitDifferenceSort(a):
diff =
for i in a:
i = list(str(i))
diff.append(i)
for i in range(len(diff)):
for j in range(i+1, len(diff)):
if int(max(diff[i])) - int(min(diff[i])) > int(max(diff[j])) - int(min(diff[j])):
diff[j], diff[i] = diff[i], diff[j]
elif int(max(diff[i])) - int(min(diff[i])) == int(max(diff[j])) - int(min(diff[j])):
diff[i], diff[j] = diff[j], diff[i]
new_list =
for i in diff:
b = ''
for j in i:
b = b + j
new_list.append(int(b))
return new_list
python python-3.x time-limit-exceeded
python python-3.x time-limit-exceeded
edited Mar 11 at 11:12


Vogel612♦
21.9k547131
21.9k547131
asked Mar 11 at 10:01


Ibrahim RahimiIbrahim Rahimi
1005
1005
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
$begingroup$
Python has a built-in sorted
function, you should use it. What it needs to sort according to some special criteria is a key
function:
def max_digit_diff(n):
n_str = str(n)
return int(max(n_str)) - int(min(n_str))
This uses the fact that "0" < "1" < ... < "9"
.
However, the sorted
function uses a stable sorting algorithm, so if two elements compare equal, the original order is preserved. But here we want the opposite order (later elements come first), so we just reverse the list first:
def digit_difference_sort(a):
return sorted(reversed(a), key=max_digit_diff)
This should be vastly easier to read than your convoluted function. Note that the function names also follow Python's official style-guide, PEP8.
Like all (good) sorting functions, this is $mathcal{O}(n log n)$. Here is a timing comparison to your function with arrays up to length 10k (at which point your function takes more than a minute...).
Here is an implementation of the radix sort suggested by @JollyJoker in their answer:
from itertools import chain
def radix_sort(a):
sub_a = [ for _ in range(10)]
for x in a:
sub_a[max_digit_diff(x)].append(x)
return list(chain.from_iterable(reversed(x) for x in sub_a))
This seems to have the same complexity as my approach, probably the implementation of max_digit_diff
actually dominates this:
$endgroup$
$begingroup$
Thanks a lot. Would you please give more clarification about passing max_digit_diff method as a parameter to sorted function and how this part works?
$endgroup$
– Ibrahim Rahimi
Mar 11 at 10:57
1
$begingroup$
@IbrahimRahimi:sorted
calls the function you specify as akey
exactly once for each input and sorts according to that. Have a look at the official Sorting HOW TO for more information.
$endgroup$
– Graipher
Mar 11 at 11:00
1
$begingroup$
Nice answer. Just curious, How did you generate the comparison graph? is there a package for doing it?
$endgroup$
– gustavovelascoh
Mar 11 at 12:30
1
$begingroup$
@gustavovelascoh: It is basically done with the code in my question here, with some input from the answers. One of these days I will finally make it look pretty and upload it to github...
$endgroup$
– Graipher
Mar 11 at 13:56
add a comment |
$begingroup$
You can do better than $mathcal{O}(n log n)$ using a Radix sort.
The differences can only have values 0-9, so you can sort the original array into a list of 10 lists while just going through the array once. Then, for each list 0-9, pop()
the values into an output list until the list is empty.
$endgroup$
$begingroup$
Added an implementation of this to my answer and included it in the timings. Interestingly it is exactly the same as using the built-insorted
. Probably due to the fact that both usemax_digit_diff
.
$endgroup$
– Graipher
Mar 11 at 14:30
$begingroup$
@Graipher The scaling seems odd. Could you add some timing check before the return row just to check there's nothing slow in the last line? Then again, maybesorted
just is that good.
$endgroup$
– JollyJoker
Mar 11 at 15:02
$begingroup$
Weirdly, it looks exactly the same when directly returningsub_a
. Also,max_digit_diff
is basically constant time, obviously, since even the longest numbers have only a few digits (less than a hundred).
$endgroup$
– Graipher
Mar 11 at 15:18
$begingroup$
I also tried arrays with up to 10k elements, still the same (without the OPs algorithm, obviously).
$endgroup$
– Graipher
Mar 11 at 15:27
1
$begingroup$
@Graipher You're probably right on Timsort. BTW, good job on turning my answer into actual code :) I wasn't at all certain my text was clear enough.
$endgroup$
– JollyJoker
Mar 11 at 15:48
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f215180%2flist-elements-digit-difference-sort%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
Python has a built-in sorted
function, you should use it. What it needs to sort according to some special criteria is a key
function:
def max_digit_diff(n):
n_str = str(n)
return int(max(n_str)) - int(min(n_str))
This uses the fact that "0" < "1" < ... < "9"
.
However, the sorted
function uses a stable sorting algorithm, so if two elements compare equal, the original order is preserved. But here we want the opposite order (later elements come first), so we just reverse the list first:
def digit_difference_sort(a):
return sorted(reversed(a), key=max_digit_diff)
This should be vastly easier to read than your convoluted function. Note that the function names also follow Python's official style-guide, PEP8.
Like all (good) sorting functions, this is $mathcal{O}(n log n)$. Here is a timing comparison to your function with arrays up to length 10k (at which point your function takes more than a minute...).
Here is an implementation of the radix sort suggested by @JollyJoker in their answer:
from itertools import chain
def radix_sort(a):
sub_a = [ for _ in range(10)]
for x in a:
sub_a[max_digit_diff(x)].append(x)
return list(chain.from_iterable(reversed(x) for x in sub_a))
This seems to have the same complexity as my approach, probably the implementation of max_digit_diff
actually dominates this:
$endgroup$
$begingroup$
Thanks a lot. Would you please give more clarification about passing max_digit_diff method as a parameter to sorted function and how this part works?
$endgroup$
– Ibrahim Rahimi
Mar 11 at 10:57
1
$begingroup$
@IbrahimRahimi:sorted
calls the function you specify as akey
exactly once for each input and sorts according to that. Have a look at the official Sorting HOW TO for more information.
$endgroup$
– Graipher
Mar 11 at 11:00
1
$begingroup$
Nice answer. Just curious, How did you generate the comparison graph? is there a package for doing it?
$endgroup$
– gustavovelascoh
Mar 11 at 12:30
1
$begingroup$
@gustavovelascoh: It is basically done with the code in my question here, with some input from the answers. One of these days I will finally make it look pretty and upload it to github...
$endgroup$
– Graipher
Mar 11 at 13:56
add a comment |
$begingroup$
Python has a built-in sorted
function, you should use it. What it needs to sort according to some special criteria is a key
function:
def max_digit_diff(n):
n_str = str(n)
return int(max(n_str)) - int(min(n_str))
This uses the fact that "0" < "1" < ... < "9"
.
However, the sorted
function uses a stable sorting algorithm, so if two elements compare equal, the original order is preserved. But here we want the opposite order (later elements come first), so we just reverse the list first:
def digit_difference_sort(a):
return sorted(reversed(a), key=max_digit_diff)
This should be vastly easier to read than your convoluted function. Note that the function names also follow Python's official style-guide, PEP8.
Like all (good) sorting functions, this is $mathcal{O}(n log n)$. Here is a timing comparison to your function with arrays up to length 10k (at which point your function takes more than a minute...).
Here is an implementation of the radix sort suggested by @JollyJoker in their answer:
from itertools import chain
def radix_sort(a):
sub_a = [ for _ in range(10)]
for x in a:
sub_a[max_digit_diff(x)].append(x)
return list(chain.from_iterable(reversed(x) for x in sub_a))
This seems to have the same complexity as my approach, probably the implementation of max_digit_diff
actually dominates this:
$endgroup$
$begingroup$
Thanks a lot. Would you please give more clarification about passing max_digit_diff method as a parameter to sorted function and how this part works?
$endgroup$
– Ibrahim Rahimi
Mar 11 at 10:57
1
$begingroup$
@IbrahimRahimi:sorted
calls the function you specify as akey
exactly once for each input and sorts according to that. Have a look at the official Sorting HOW TO for more information.
$endgroup$
– Graipher
Mar 11 at 11:00
1
$begingroup$
Nice answer. Just curious, How did you generate the comparison graph? is there a package for doing it?
$endgroup$
– gustavovelascoh
Mar 11 at 12:30
1
$begingroup$
@gustavovelascoh: It is basically done with the code in my question here, with some input from the answers. One of these days I will finally make it look pretty and upload it to github...
$endgroup$
– Graipher
Mar 11 at 13:56
add a comment |
$begingroup$
Python has a built-in sorted
function, you should use it. What it needs to sort according to some special criteria is a key
function:
def max_digit_diff(n):
n_str = str(n)
return int(max(n_str)) - int(min(n_str))
This uses the fact that "0" < "1" < ... < "9"
.
However, the sorted
function uses a stable sorting algorithm, so if two elements compare equal, the original order is preserved. But here we want the opposite order (later elements come first), so we just reverse the list first:
def digit_difference_sort(a):
return sorted(reversed(a), key=max_digit_diff)
This should be vastly easier to read than your convoluted function. Note that the function names also follow Python's official style-guide, PEP8.
Like all (good) sorting functions, this is $mathcal{O}(n log n)$. Here is a timing comparison to your function with arrays up to length 10k (at which point your function takes more than a minute...).
Here is an implementation of the radix sort suggested by @JollyJoker in their answer:
from itertools import chain
def radix_sort(a):
sub_a = [ for _ in range(10)]
for x in a:
sub_a[max_digit_diff(x)].append(x)
return list(chain.from_iterable(reversed(x) for x in sub_a))
This seems to have the same complexity as my approach, probably the implementation of max_digit_diff
actually dominates this:
$endgroup$
Python has a built-in sorted
function, you should use it. What it needs to sort according to some special criteria is a key
function:
def max_digit_diff(n):
n_str = str(n)
return int(max(n_str)) - int(min(n_str))
This uses the fact that "0" < "1" < ... < "9"
.
However, the sorted
function uses a stable sorting algorithm, so if two elements compare equal, the original order is preserved. But here we want the opposite order (later elements come first), so we just reverse the list first:
def digit_difference_sort(a):
return sorted(reversed(a), key=max_digit_diff)
This should be vastly easier to read than your convoluted function. Note that the function names also follow Python's official style-guide, PEP8.
Like all (good) sorting functions, this is $mathcal{O}(n log n)$. Here is a timing comparison to your function with arrays up to length 10k (at which point your function takes more than a minute...).
Here is an implementation of the radix sort suggested by @JollyJoker in their answer:
from itertools import chain
def radix_sort(a):
sub_a = [ for _ in range(10)]
for x in a:
sub_a[max_digit_diff(x)].append(x)
return list(chain.from_iterable(reversed(x) for x in sub_a))
This seems to have the same complexity as my approach, probably the implementation of max_digit_diff
actually dominates this:
edited Mar 11 at 14:29
answered Mar 11 at 10:30
GraipherGraipher
27.2k54497
27.2k54497
$begingroup$
Thanks a lot. Would you please give more clarification about passing max_digit_diff method as a parameter to sorted function and how this part works?
$endgroup$
– Ibrahim Rahimi
Mar 11 at 10:57
1
$begingroup$
@IbrahimRahimi:sorted
calls the function you specify as akey
exactly once for each input and sorts according to that. Have a look at the official Sorting HOW TO for more information.
$endgroup$
– Graipher
Mar 11 at 11:00
1
$begingroup$
Nice answer. Just curious, How did you generate the comparison graph? is there a package for doing it?
$endgroup$
– gustavovelascoh
Mar 11 at 12:30
1
$begingroup$
@gustavovelascoh: It is basically done with the code in my question here, with some input from the answers. One of these days I will finally make it look pretty and upload it to github...
$endgroup$
– Graipher
Mar 11 at 13:56
add a comment |
$begingroup$
Thanks a lot. Would you please give more clarification about passing max_digit_diff method as a parameter to sorted function and how this part works?
$endgroup$
– Ibrahim Rahimi
Mar 11 at 10:57
1
$begingroup$
@IbrahimRahimi:sorted
calls the function you specify as akey
exactly once for each input and sorts according to that. Have a look at the official Sorting HOW TO for more information.
$endgroup$
– Graipher
Mar 11 at 11:00
1
$begingroup$
Nice answer. Just curious, How did you generate the comparison graph? is there a package for doing it?
$endgroup$
– gustavovelascoh
Mar 11 at 12:30
1
$begingroup$
@gustavovelascoh: It is basically done with the code in my question here, with some input from the answers. One of these days I will finally make it look pretty and upload it to github...
$endgroup$
– Graipher
Mar 11 at 13:56
$begingroup$
Thanks a lot. Would you please give more clarification about passing max_digit_diff method as a parameter to sorted function and how this part works?
$endgroup$
– Ibrahim Rahimi
Mar 11 at 10:57
$begingroup$
Thanks a lot. Would you please give more clarification about passing max_digit_diff method as a parameter to sorted function and how this part works?
$endgroup$
– Ibrahim Rahimi
Mar 11 at 10:57
1
1
$begingroup$
@IbrahimRahimi:
sorted
calls the function you specify as a key
exactly once for each input and sorts according to that. Have a look at the official Sorting HOW TO for more information.$endgroup$
– Graipher
Mar 11 at 11:00
$begingroup$
@IbrahimRahimi:
sorted
calls the function you specify as a key
exactly once for each input and sorts according to that. Have a look at the official Sorting HOW TO for more information.$endgroup$
– Graipher
Mar 11 at 11:00
1
1
$begingroup$
Nice answer. Just curious, How did you generate the comparison graph? is there a package for doing it?
$endgroup$
– gustavovelascoh
Mar 11 at 12:30
$begingroup$
Nice answer. Just curious, How did you generate the comparison graph? is there a package for doing it?
$endgroup$
– gustavovelascoh
Mar 11 at 12:30
1
1
$begingroup$
@gustavovelascoh: It is basically done with the code in my question here, with some input from the answers. One of these days I will finally make it look pretty and upload it to github...
$endgroup$
– Graipher
Mar 11 at 13:56
$begingroup$
@gustavovelascoh: It is basically done with the code in my question here, with some input from the answers. One of these days I will finally make it look pretty and upload it to github...
$endgroup$
– Graipher
Mar 11 at 13:56
add a comment |
$begingroup$
You can do better than $mathcal{O}(n log n)$ using a Radix sort.
The differences can only have values 0-9, so you can sort the original array into a list of 10 lists while just going through the array once. Then, for each list 0-9, pop()
the values into an output list until the list is empty.
$endgroup$
$begingroup$
Added an implementation of this to my answer and included it in the timings. Interestingly it is exactly the same as using the built-insorted
. Probably due to the fact that both usemax_digit_diff
.
$endgroup$
– Graipher
Mar 11 at 14:30
$begingroup$
@Graipher The scaling seems odd. Could you add some timing check before the return row just to check there's nothing slow in the last line? Then again, maybesorted
just is that good.
$endgroup$
– JollyJoker
Mar 11 at 15:02
$begingroup$
Weirdly, it looks exactly the same when directly returningsub_a
. Also,max_digit_diff
is basically constant time, obviously, since even the longest numbers have only a few digits (less than a hundred).
$endgroup$
– Graipher
Mar 11 at 15:18
$begingroup$
I also tried arrays with up to 10k elements, still the same (without the OPs algorithm, obviously).
$endgroup$
– Graipher
Mar 11 at 15:27
1
$begingroup$
@Graipher You're probably right on Timsort. BTW, good job on turning my answer into actual code :) I wasn't at all certain my text was clear enough.
$endgroup$
– JollyJoker
Mar 11 at 15:48
|
show 1 more comment
$begingroup$
You can do better than $mathcal{O}(n log n)$ using a Radix sort.
The differences can only have values 0-9, so you can sort the original array into a list of 10 lists while just going through the array once. Then, for each list 0-9, pop()
the values into an output list until the list is empty.
$endgroup$
$begingroup$
Added an implementation of this to my answer and included it in the timings. Interestingly it is exactly the same as using the built-insorted
. Probably due to the fact that both usemax_digit_diff
.
$endgroup$
– Graipher
Mar 11 at 14:30
$begingroup$
@Graipher The scaling seems odd. Could you add some timing check before the return row just to check there's nothing slow in the last line? Then again, maybesorted
just is that good.
$endgroup$
– JollyJoker
Mar 11 at 15:02
$begingroup$
Weirdly, it looks exactly the same when directly returningsub_a
. Also,max_digit_diff
is basically constant time, obviously, since even the longest numbers have only a few digits (less than a hundred).
$endgroup$
– Graipher
Mar 11 at 15:18
$begingroup$
I also tried arrays with up to 10k elements, still the same (without the OPs algorithm, obviously).
$endgroup$
– Graipher
Mar 11 at 15:27
1
$begingroup$
@Graipher You're probably right on Timsort. BTW, good job on turning my answer into actual code :) I wasn't at all certain my text was clear enough.
$endgroup$
– JollyJoker
Mar 11 at 15:48
|
show 1 more comment
$begingroup$
You can do better than $mathcal{O}(n log n)$ using a Radix sort.
The differences can only have values 0-9, so you can sort the original array into a list of 10 lists while just going through the array once. Then, for each list 0-9, pop()
the values into an output list until the list is empty.
$endgroup$
You can do better than $mathcal{O}(n log n)$ using a Radix sort.
The differences can only have values 0-9, so you can sort the original array into a list of 10 lists while just going through the array once. Then, for each list 0-9, pop()
the values into an output list until the list is empty.
answered Mar 11 at 14:11
JollyJokerJollyJoker
36116
36116
$begingroup$
Added an implementation of this to my answer and included it in the timings. Interestingly it is exactly the same as using the built-insorted
. Probably due to the fact that both usemax_digit_diff
.
$endgroup$
– Graipher
Mar 11 at 14:30
$begingroup$
@Graipher The scaling seems odd. Could you add some timing check before the return row just to check there's nothing slow in the last line? Then again, maybesorted
just is that good.
$endgroup$
– JollyJoker
Mar 11 at 15:02
$begingroup$
Weirdly, it looks exactly the same when directly returningsub_a
. Also,max_digit_diff
is basically constant time, obviously, since even the longest numbers have only a few digits (less than a hundred).
$endgroup$
– Graipher
Mar 11 at 15:18
$begingroup$
I also tried arrays with up to 10k elements, still the same (without the OPs algorithm, obviously).
$endgroup$
– Graipher
Mar 11 at 15:27
1
$begingroup$
@Graipher You're probably right on Timsort. BTW, good job on turning my answer into actual code :) I wasn't at all certain my text was clear enough.
$endgroup$
– JollyJoker
Mar 11 at 15:48
|
show 1 more comment
$begingroup$
Added an implementation of this to my answer and included it in the timings. Interestingly it is exactly the same as using the built-insorted
. Probably due to the fact that both usemax_digit_diff
.
$endgroup$
– Graipher
Mar 11 at 14:30
$begingroup$
@Graipher The scaling seems odd. Could you add some timing check before the return row just to check there's nothing slow in the last line? Then again, maybesorted
just is that good.
$endgroup$
– JollyJoker
Mar 11 at 15:02
$begingroup$
Weirdly, it looks exactly the same when directly returningsub_a
. Also,max_digit_diff
is basically constant time, obviously, since even the longest numbers have only a few digits (less than a hundred).
$endgroup$
– Graipher
Mar 11 at 15:18
$begingroup$
I also tried arrays with up to 10k elements, still the same (without the OPs algorithm, obviously).
$endgroup$
– Graipher
Mar 11 at 15:27
1
$begingroup$
@Graipher You're probably right on Timsort. BTW, good job on turning my answer into actual code :) I wasn't at all certain my text was clear enough.
$endgroup$
– JollyJoker
Mar 11 at 15:48
$begingroup$
Added an implementation of this to my answer and included it in the timings. Interestingly it is exactly the same as using the built-in
sorted
. Probably due to the fact that both use max_digit_diff
.$endgroup$
– Graipher
Mar 11 at 14:30
$begingroup$
Added an implementation of this to my answer and included it in the timings. Interestingly it is exactly the same as using the built-in
sorted
. Probably due to the fact that both use max_digit_diff
.$endgroup$
– Graipher
Mar 11 at 14:30
$begingroup$
@Graipher The scaling seems odd. Could you add some timing check before the return row just to check there's nothing slow in the last line? Then again, maybe
sorted
just is that good.$endgroup$
– JollyJoker
Mar 11 at 15:02
$begingroup$
@Graipher The scaling seems odd. Could you add some timing check before the return row just to check there's nothing slow in the last line? Then again, maybe
sorted
just is that good.$endgroup$
– JollyJoker
Mar 11 at 15:02
$begingroup$
Weirdly, it looks exactly the same when directly returning
sub_a
. Also, max_digit_diff
is basically constant time, obviously, since even the longest numbers have only a few digits (less than a hundred).$endgroup$
– Graipher
Mar 11 at 15:18
$begingroup$
Weirdly, it looks exactly the same when directly returning
sub_a
. Also, max_digit_diff
is basically constant time, obviously, since even the longest numbers have only a few digits (less than a hundred).$endgroup$
– Graipher
Mar 11 at 15:18
$begingroup$
I also tried arrays with up to 10k elements, still the same (without the OPs algorithm, obviously).
$endgroup$
– Graipher
Mar 11 at 15:27
$begingroup$
I also tried arrays with up to 10k elements, still the same (without the OPs algorithm, obviously).
$endgroup$
– Graipher
Mar 11 at 15:27
1
1
$begingroup$
@Graipher You're probably right on Timsort. BTW, good job on turning my answer into actual code :) I wasn't at all certain my text was clear enough.
$endgroup$
– JollyJoker
Mar 11 at 15:48
$begingroup$
@Graipher You're probably right on Timsort. BTW, good job on turning my answer into actual code :) I wasn't at all certain my text was clear enough.
$endgroup$
– JollyJoker
Mar 11 at 15:48
|
show 1 more comment
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f215180%2flist-elements-digit-difference-sort%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bMu,5vNelo SnV8sTwN5vOdqY 0 9wwLH5eysS0JyxP uC1lbO X6 On JJr9SSyz6atJAJg7O7YXO8DIqdvOouRWMbEKg