How to receive standard input from keyboad properly in a child process?
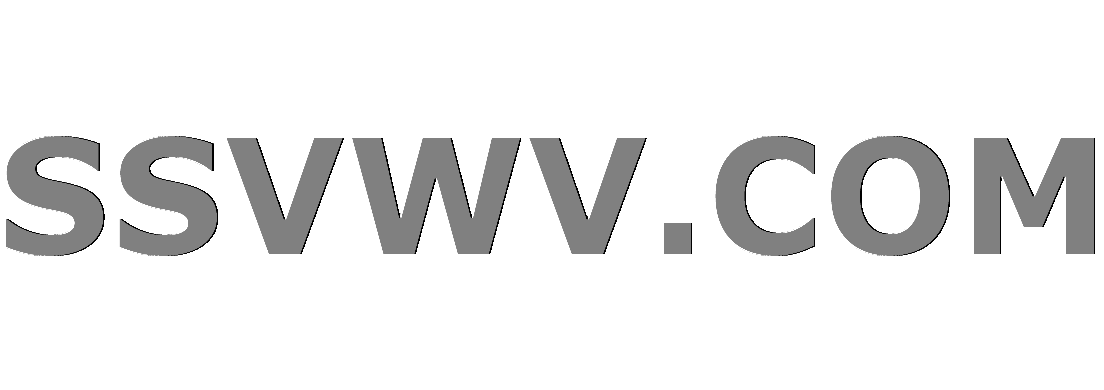
Multi tool use
I'm running the following C program on Linux.
Program
// program.c
#include <stdio.h>
#include <unistd.h>
int main() {
if (fork() == 0) { // child process
int a, b;
scanf("%d %d", &a, &b);
printf("%d + %d = %dn", a, b, a + b);
}
return 0;
}
Expected behavior
$ ./program
1 2
1 + 2 = 3
Actual behavior
$ ./program
$ 1222245440 + 32764 = 1222278204
When I run the program in the terminal, the program will soon produce strange output like 1222245440 + 32764 = 1222278204
and doesn't wait for me to type. I think the problem is that the standard input and output streams of the child process are not attached to the terminal. Instead, the streams of parent process are attached. As a result, the child process cannot get my input from keyboard. Is there a method to solve this problem? I mean, to detach the streams of parent process and attach the streams of child process. For some reason, I must receive inputs in the child process.
linux bash terminal c
migrated from superuser.com Feb 26 at 12:45
This question came from our site for computer enthusiasts and power users.
add a comment |
I'm running the following C program on Linux.
Program
// program.c
#include <stdio.h>
#include <unistd.h>
int main() {
if (fork() == 0) { // child process
int a, b;
scanf("%d %d", &a, &b);
printf("%d + %d = %dn", a, b, a + b);
}
return 0;
}
Expected behavior
$ ./program
1 2
1 + 2 = 3
Actual behavior
$ ./program
$ 1222245440 + 32764 = 1222278204
When I run the program in the terminal, the program will soon produce strange output like 1222245440 + 32764 = 1222278204
and doesn't wait for me to type. I think the problem is that the standard input and output streams of the child process are not attached to the terminal. Instead, the streams of parent process are attached. As a result, the child process cannot get my input from keyboard. Is there a method to solve this problem? I mean, to detach the streams of parent process and attach the streams of child process. For some reason, I must receive inputs in the child process.
linux bash terminal c
migrated from superuser.com Feb 26 at 12:45
This question came from our site for computer enthusiasts and power users.
add a comment |
I'm running the following C program on Linux.
Program
// program.c
#include <stdio.h>
#include <unistd.h>
int main() {
if (fork() == 0) { // child process
int a, b;
scanf("%d %d", &a, &b);
printf("%d + %d = %dn", a, b, a + b);
}
return 0;
}
Expected behavior
$ ./program
1 2
1 + 2 = 3
Actual behavior
$ ./program
$ 1222245440 + 32764 = 1222278204
When I run the program in the terminal, the program will soon produce strange output like 1222245440 + 32764 = 1222278204
and doesn't wait for me to type. I think the problem is that the standard input and output streams of the child process are not attached to the terminal. Instead, the streams of parent process are attached. As a result, the child process cannot get my input from keyboard. Is there a method to solve this problem? I mean, to detach the streams of parent process and attach the streams of child process. For some reason, I must receive inputs in the child process.
linux bash terminal c
I'm running the following C program on Linux.
Program
// program.c
#include <stdio.h>
#include <unistd.h>
int main() {
if (fork() == 0) { // child process
int a, b;
scanf("%d %d", &a, &b);
printf("%d + %d = %dn", a, b, a + b);
}
return 0;
}
Expected behavior
$ ./program
1 2
1 + 2 = 3
Actual behavior
$ ./program
$ 1222245440 + 32764 = 1222278204
When I run the program in the terminal, the program will soon produce strange output like 1222245440 + 32764 = 1222278204
and doesn't wait for me to type. I think the problem is that the standard input and output streams of the child process are not attached to the terminal. Instead, the streams of parent process are attached. As a result, the child process cannot get my input from keyboard. Is there a method to solve this problem? I mean, to detach the streams of parent process and attach the streams of child process. For some reason, I must receive inputs in the child process.
linux bash terminal c
linux bash terminal c
asked Feb 19 at 7:59
Wang TianzeWang Tianze
367
367
migrated from superuser.com Feb 26 at 12:45
This question came from our site for computer enthusiasts and power users.
migrated from superuser.com Feb 26 at 12:45
This question came from our site for computer enthusiasts and power users.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I think the problem is that the standard input and output streams of the child process are not attached to the terminal. Instead, the streams of parent process are attached.
The parent process doesn't wait for the child and returns immediately. The orphaned child cannot read from the terminal, the "strange output" is from garbage values of a
and b
. See what scanf
returns, run this code:
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
int wstatus;
if (fork() == 0) { // child process
int a, b, n;
n=scanf("%d %d", &a, &b);
printf("(%d returned) %d + %d = %dn", n, a, b, a + b);
}
// wait(&wstatus);
return 0;
}
You will most likely get something like (-1 returned) 1222245440 + 32764 = 1222278204
. Then uncomment wait
and try again.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54885900%2fhow-to-receive-standard-input-from-keyboad-properly-in-a-child-process%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think the problem is that the standard input and output streams of the child process are not attached to the terminal. Instead, the streams of parent process are attached.
The parent process doesn't wait for the child and returns immediately. The orphaned child cannot read from the terminal, the "strange output" is from garbage values of a
and b
. See what scanf
returns, run this code:
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
int wstatus;
if (fork() == 0) { // child process
int a, b, n;
n=scanf("%d %d", &a, &b);
printf("(%d returned) %d + %d = %dn", n, a, b, a + b);
}
// wait(&wstatus);
return 0;
}
You will most likely get something like (-1 returned) 1222245440 + 32764 = 1222278204
. Then uncomment wait
and try again.
add a comment |
I think the problem is that the standard input and output streams of the child process are not attached to the terminal. Instead, the streams of parent process are attached.
The parent process doesn't wait for the child and returns immediately. The orphaned child cannot read from the terminal, the "strange output" is from garbage values of a
and b
. See what scanf
returns, run this code:
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
int wstatus;
if (fork() == 0) { // child process
int a, b, n;
n=scanf("%d %d", &a, &b);
printf("(%d returned) %d + %d = %dn", n, a, b, a + b);
}
// wait(&wstatus);
return 0;
}
You will most likely get something like (-1 returned) 1222245440 + 32764 = 1222278204
. Then uncomment wait
and try again.
add a comment |
I think the problem is that the standard input and output streams of the child process are not attached to the terminal. Instead, the streams of parent process are attached.
The parent process doesn't wait for the child and returns immediately. The orphaned child cannot read from the terminal, the "strange output" is from garbage values of a
and b
. See what scanf
returns, run this code:
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
int wstatus;
if (fork() == 0) { // child process
int a, b, n;
n=scanf("%d %d", &a, &b);
printf("(%d returned) %d + %d = %dn", n, a, b, a + b);
}
// wait(&wstatus);
return 0;
}
You will most likely get something like (-1 returned) 1222245440 + 32764 = 1222278204
. Then uncomment wait
and try again.
I think the problem is that the standard input and output streams of the child process are not attached to the terminal. Instead, the streams of parent process are attached.
The parent process doesn't wait for the child and returns immediately. The orphaned child cannot read from the terminal, the "strange output" is from garbage values of a
and b
. See what scanf
returns, run this code:
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
int wstatus;
if (fork() == 0) { // child process
int a, b, n;
n=scanf("%d %d", &a, &b);
printf("(%d returned) %d + %d = %dn", n, a, b, a + b);
}
// wait(&wstatus);
return 0;
}
You will most likely get something like (-1 returned) 1222245440 + 32764 = 1222278204
. Then uncomment wait
and try again.
answered Feb 19 at 9:31
Kamil MaciorowskiKamil Maciorowski
2006
2006
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54885900%2fhow-to-receive-standard-input-from-keyboad-properly-in-a-child-process%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
wiQ7oAjkh5d,gCk,NyoD,Yms131Dh69