Trying to push an unknown number of strings into a vector, but my cin loop doesn't terminate [closed]
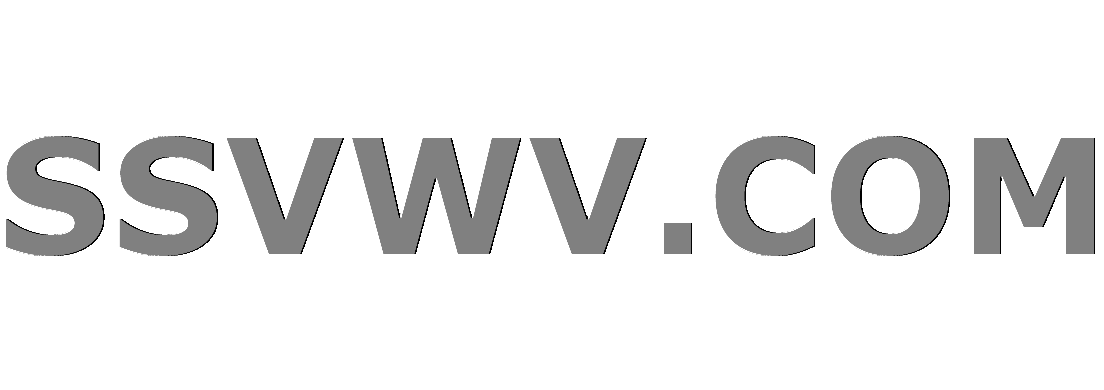
Multi tool use
I am trying to take strings as input from cin
, and then push the string into a vector each time. However, my loop doesn't terminate even when I put a '' at the end of all my input.
int main(void) {
string row;
vector<string> log;
while (cin >> row) {
if (row == "n") {
break;
}
log.push_back(row);
}
return 0;
}
I've tried replacing the (cin >> row
) with (getline(cin,row)
), but it didn't make any difference. I've tried using stringstream, but I don't really know how it works. How do I go about resolving this?
c++ cin
closed as off-topic by Sid S, gsamaras, Umair, G.hakim, Bob Dalgleish Jan 23 at 14:10
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This question was caused by a problem that can no longer be reproduced or a simple typographical error. While similar questions may be on-topic here, this one was resolved in a manner unlikely to help future readers. This can often be avoided by identifying and closely inspecting the shortest program necessary to reproduce the problem before posting." – Sid S, gsamaras, Umair, G.hakim, Bob Dalgleish
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
I am trying to take strings as input from cin
, and then push the string into a vector each time. However, my loop doesn't terminate even when I put a '' at the end of all my input.
int main(void) {
string row;
vector<string> log;
while (cin >> row) {
if (row == "n") {
break;
}
log.push_back(row);
}
return 0;
}
I've tried replacing the (cin >> row
) with (getline(cin,row)
), but it didn't make any difference. I've tried using stringstream, but I don't really know how it works. How do I go about resolving this?
c++ cin
closed as off-topic by Sid S, gsamaras, Umair, G.hakim, Bob Dalgleish Jan 23 at 14:10
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This question was caused by a problem that can no longer be reproduced or a simple typographical error. While similar questions may be on-topic here, this one was resolved in a manner unlikely to help future readers. This can often be avoided by identifying and closely inspecting the shortest program necessary to reproduce the problem before posting." – Sid S, gsamaras, Umair, G.hakim, Bob Dalgleish
If this question can be reworded to fit the rules in the help center, please edit the question.
8
cin >> row
discards whitespace, sorow
is never going to be"n"
. If you want to end on , break onrow == "\"
.
– Sid S
Jan 23 at 7:55
3
Also consider breaking onrow.empty()
.
– Sid S
Jan 23 at 8:02
add a comment |
I am trying to take strings as input from cin
, and then push the string into a vector each time. However, my loop doesn't terminate even when I put a '' at the end of all my input.
int main(void) {
string row;
vector<string> log;
while (cin >> row) {
if (row == "n") {
break;
}
log.push_back(row);
}
return 0;
}
I've tried replacing the (cin >> row
) with (getline(cin,row)
), but it didn't make any difference. I've tried using stringstream, but I don't really know how it works. How do I go about resolving this?
c++ cin
I am trying to take strings as input from cin
, and then push the string into a vector each time. However, my loop doesn't terminate even when I put a '' at the end of all my input.
int main(void) {
string row;
vector<string> log;
while (cin >> row) {
if (row == "n") {
break;
}
log.push_back(row);
}
return 0;
}
I've tried replacing the (cin >> row
) with (getline(cin,row)
), but it didn't make any difference. I've tried using stringstream, but I don't really know how it works. How do I go about resolving this?
c++ cin
c++ cin
edited Jan 23 at 8:01
Ivaylo Strandjev
56.5k1086142
56.5k1086142
asked Jan 23 at 7:53
cluelessguycluelessguy
461
461
closed as off-topic by Sid S, gsamaras, Umair, G.hakim, Bob Dalgleish Jan 23 at 14:10
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This question was caused by a problem that can no longer be reproduced or a simple typographical error. While similar questions may be on-topic here, this one was resolved in a manner unlikely to help future readers. This can often be avoided by identifying and closely inspecting the shortest program necessary to reproduce the problem before posting." – Sid S, gsamaras, Umair, G.hakim, Bob Dalgleish
If this question can be reworded to fit the rules in the help center, please edit the question.
closed as off-topic by Sid S, gsamaras, Umair, G.hakim, Bob Dalgleish Jan 23 at 14:10
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This question was caused by a problem that can no longer be reproduced or a simple typographical error. While similar questions may be on-topic here, this one was resolved in a manner unlikely to help future readers. This can often be avoided by identifying and closely inspecting the shortest program necessary to reproduce the problem before posting." – Sid S, gsamaras, Umair, G.hakim, Bob Dalgleish
If this question can be reworded to fit the rules in the help center, please edit the question.
8
cin >> row
discards whitespace, sorow
is never going to be"n"
. If you want to end on , break onrow == "\"
.
– Sid S
Jan 23 at 7:55
3
Also consider breaking onrow.empty()
.
– Sid S
Jan 23 at 8:02
add a comment |
8
cin >> row
discards whitespace, sorow
is never going to be"n"
. If you want to end on , break onrow == "\"
.
– Sid S
Jan 23 at 7:55
3
Also consider breaking onrow.empty()
.
– Sid S
Jan 23 at 8:02
8
8
cin >> row
discards whitespace, so row
is never going to be "n"
. If you want to end on , break on row == "\"
.– Sid S
Jan 23 at 7:55
cin >> row
discards whitespace, so row
is never going to be "n"
. If you want to end on , break on row == "\"
.– Sid S
Jan 23 at 7:55
3
3
Also consider breaking on
row.empty()
.– Sid S
Jan 23 at 8:02
Also consider breaking on
row.empty()
.– Sid S
Jan 23 at 8:02
add a comment |
3 Answers
3
active
oldest
votes
As commented by @SidS, the whitespace is discarded. So you have to think about another strategy.
You could instead check if row
is empty. But that will only work with std::getline
:
#include <vector>
#include <string>
#include <iostream>
int main() {
std::string row;
std::vector<std::string> log;
while (std::getline(std::cin, row)) {
if (row.empty()) {
break;
}
log.push_back(row);
}
std::cout << "donen";
}
OP, in case you want to save single words (rather than a whole line), you can use regex
to single-handedly push each of them into row
after input:
#include <vector>
#include <string>
#include <iostream>
#include <regex>
int main() {
const std::regex words_reg{ "[^\s]+" };
std::string row;
std::vector<std::string> log;
while (std::getline(std::cin, row)) {
if (row.empty()) {
break;
}
for (auto it = std::sregex_iterator(row.begin(), row.end(), words_reg); it != std::sregex_iterator(); ++it){
log.push_back((*it)[0]);
}
}
for (unsigned i = 0u; i < log.size(); ++i) {
std::cout << "log[" << i << "] = " << log[i] << 'n';
}
}
Example run:
hello you
a b c d e f g
18939823
@_@_@ /////
log[0] = hello
log[1] = you
log[2] = a
log[3] = b
log[4] = c
log[5] = d
log[6] = e
log[7] = f
log[8] = g
log[9] = 18939823
log[10] = @_@_@
log[11] = /////
Isn't the OP's original goal to store an individual token as astd::string
in the container, rather than each line? There should be an additional step to split each row according to a given delimiter.
– lubgr
Jan 23 at 8:06
@lubgr yeah you're right, I will add some regex for the curious, too
– Stack Danny
Jan 23 at 8:11
@lubgr in fact I am not certain what is the original intent of the OP, as the variable name is row I think storing the whole line is more plausible
– Ivaylo Strandjev
Jan 23 at 8:14
add a comment |
If you want to store the tokens of one line from std::cin
, separated by the standard mechanism as in the operator>>
overloads from <iostream>
(i.e., split by whitespace/newline), you can do it like this:
std::string line;
std::getline(std::cin, line);
std::stringstream ss{line};
const std::vector<std::string> tokens{std::istream_iterator<std::string>{ss},
std::istream_iterator<std::string>{}};
Note that this is not the most efficient solution, but it should work as expected: process only one line and use an existing mechanism to split this line into individual std::string
objects.
add a comment |
You can't read newline by using the istream& operator >>
of string. This operator ignores whitespaces and will never return the string "n"
. Consider using getline instead.
getline()
won't return"n"
either.
– Sid S
Jan 23 at 8:26
1
No, it will not but it will serve the purpose to read a whole row.
– Ivaylo Strandjev
Jan 23 at 8:30
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
As commented by @SidS, the whitespace is discarded. So you have to think about another strategy.
You could instead check if row
is empty. But that will only work with std::getline
:
#include <vector>
#include <string>
#include <iostream>
int main() {
std::string row;
std::vector<std::string> log;
while (std::getline(std::cin, row)) {
if (row.empty()) {
break;
}
log.push_back(row);
}
std::cout << "donen";
}
OP, in case you want to save single words (rather than a whole line), you can use regex
to single-handedly push each of them into row
after input:
#include <vector>
#include <string>
#include <iostream>
#include <regex>
int main() {
const std::regex words_reg{ "[^\s]+" };
std::string row;
std::vector<std::string> log;
while (std::getline(std::cin, row)) {
if (row.empty()) {
break;
}
for (auto it = std::sregex_iterator(row.begin(), row.end(), words_reg); it != std::sregex_iterator(); ++it){
log.push_back((*it)[0]);
}
}
for (unsigned i = 0u; i < log.size(); ++i) {
std::cout << "log[" << i << "] = " << log[i] << 'n';
}
}
Example run:
hello you
a b c d e f g
18939823
@_@_@ /////
log[0] = hello
log[1] = you
log[2] = a
log[3] = b
log[4] = c
log[5] = d
log[6] = e
log[7] = f
log[8] = g
log[9] = 18939823
log[10] = @_@_@
log[11] = /////
Isn't the OP's original goal to store an individual token as astd::string
in the container, rather than each line? There should be an additional step to split each row according to a given delimiter.
– lubgr
Jan 23 at 8:06
@lubgr yeah you're right, I will add some regex for the curious, too
– Stack Danny
Jan 23 at 8:11
@lubgr in fact I am not certain what is the original intent of the OP, as the variable name is row I think storing the whole line is more plausible
– Ivaylo Strandjev
Jan 23 at 8:14
add a comment |
As commented by @SidS, the whitespace is discarded. So you have to think about another strategy.
You could instead check if row
is empty. But that will only work with std::getline
:
#include <vector>
#include <string>
#include <iostream>
int main() {
std::string row;
std::vector<std::string> log;
while (std::getline(std::cin, row)) {
if (row.empty()) {
break;
}
log.push_back(row);
}
std::cout << "donen";
}
OP, in case you want to save single words (rather than a whole line), you can use regex
to single-handedly push each of them into row
after input:
#include <vector>
#include <string>
#include <iostream>
#include <regex>
int main() {
const std::regex words_reg{ "[^\s]+" };
std::string row;
std::vector<std::string> log;
while (std::getline(std::cin, row)) {
if (row.empty()) {
break;
}
for (auto it = std::sregex_iterator(row.begin(), row.end(), words_reg); it != std::sregex_iterator(); ++it){
log.push_back((*it)[0]);
}
}
for (unsigned i = 0u; i < log.size(); ++i) {
std::cout << "log[" << i << "] = " << log[i] << 'n';
}
}
Example run:
hello you
a b c d e f g
18939823
@_@_@ /////
log[0] = hello
log[1] = you
log[2] = a
log[3] = b
log[4] = c
log[5] = d
log[6] = e
log[7] = f
log[8] = g
log[9] = 18939823
log[10] = @_@_@
log[11] = /////
Isn't the OP's original goal to store an individual token as astd::string
in the container, rather than each line? There should be an additional step to split each row according to a given delimiter.
– lubgr
Jan 23 at 8:06
@lubgr yeah you're right, I will add some regex for the curious, too
– Stack Danny
Jan 23 at 8:11
@lubgr in fact I am not certain what is the original intent of the OP, as the variable name is row I think storing the whole line is more plausible
– Ivaylo Strandjev
Jan 23 at 8:14
add a comment |
As commented by @SidS, the whitespace is discarded. So you have to think about another strategy.
You could instead check if row
is empty. But that will only work with std::getline
:
#include <vector>
#include <string>
#include <iostream>
int main() {
std::string row;
std::vector<std::string> log;
while (std::getline(std::cin, row)) {
if (row.empty()) {
break;
}
log.push_back(row);
}
std::cout << "donen";
}
OP, in case you want to save single words (rather than a whole line), you can use regex
to single-handedly push each of them into row
after input:
#include <vector>
#include <string>
#include <iostream>
#include <regex>
int main() {
const std::regex words_reg{ "[^\s]+" };
std::string row;
std::vector<std::string> log;
while (std::getline(std::cin, row)) {
if (row.empty()) {
break;
}
for (auto it = std::sregex_iterator(row.begin(), row.end(), words_reg); it != std::sregex_iterator(); ++it){
log.push_back((*it)[0]);
}
}
for (unsigned i = 0u; i < log.size(); ++i) {
std::cout << "log[" << i << "] = " << log[i] << 'n';
}
}
Example run:
hello you
a b c d e f g
18939823
@_@_@ /////
log[0] = hello
log[1] = you
log[2] = a
log[3] = b
log[4] = c
log[5] = d
log[6] = e
log[7] = f
log[8] = g
log[9] = 18939823
log[10] = @_@_@
log[11] = /////
As commented by @SidS, the whitespace is discarded. So you have to think about another strategy.
You could instead check if row
is empty. But that will only work with std::getline
:
#include <vector>
#include <string>
#include <iostream>
int main() {
std::string row;
std::vector<std::string> log;
while (std::getline(std::cin, row)) {
if (row.empty()) {
break;
}
log.push_back(row);
}
std::cout << "donen";
}
OP, in case you want to save single words (rather than a whole line), you can use regex
to single-handedly push each of them into row
after input:
#include <vector>
#include <string>
#include <iostream>
#include <regex>
int main() {
const std::regex words_reg{ "[^\s]+" };
std::string row;
std::vector<std::string> log;
while (std::getline(std::cin, row)) {
if (row.empty()) {
break;
}
for (auto it = std::sregex_iterator(row.begin(), row.end(), words_reg); it != std::sregex_iterator(); ++it){
log.push_back((*it)[0]);
}
}
for (unsigned i = 0u; i < log.size(); ++i) {
std::cout << "log[" << i << "] = " << log[i] << 'n';
}
}
Example run:
hello you
a b c d e f g
18939823
@_@_@ /////
log[0] = hello
log[1] = you
log[2] = a
log[3] = b
log[4] = c
log[5] = d
log[6] = e
log[7] = f
log[8] = g
log[9] = 18939823
log[10] = @_@_@
log[11] = /////
edited Jan 23 at 9:37
answered Jan 23 at 8:03


Stack DannyStack Danny
1,476420
1,476420
Isn't the OP's original goal to store an individual token as astd::string
in the container, rather than each line? There should be an additional step to split each row according to a given delimiter.
– lubgr
Jan 23 at 8:06
@lubgr yeah you're right, I will add some regex for the curious, too
– Stack Danny
Jan 23 at 8:11
@lubgr in fact I am not certain what is the original intent of the OP, as the variable name is row I think storing the whole line is more plausible
– Ivaylo Strandjev
Jan 23 at 8:14
add a comment |
Isn't the OP's original goal to store an individual token as astd::string
in the container, rather than each line? There should be an additional step to split each row according to a given delimiter.
– lubgr
Jan 23 at 8:06
@lubgr yeah you're right, I will add some regex for the curious, too
– Stack Danny
Jan 23 at 8:11
@lubgr in fact I am not certain what is the original intent of the OP, as the variable name is row I think storing the whole line is more plausible
– Ivaylo Strandjev
Jan 23 at 8:14
Isn't the OP's original goal to store an individual token as a
std::string
in the container, rather than each line? There should be an additional step to split each row according to a given delimiter.– lubgr
Jan 23 at 8:06
Isn't the OP's original goal to store an individual token as a
std::string
in the container, rather than each line? There should be an additional step to split each row according to a given delimiter.– lubgr
Jan 23 at 8:06
@lubgr yeah you're right, I will add some regex for the curious, too
– Stack Danny
Jan 23 at 8:11
@lubgr yeah you're right, I will add some regex for the curious, too
– Stack Danny
Jan 23 at 8:11
@lubgr in fact I am not certain what is the original intent of the OP, as the variable name is row I think storing the whole line is more plausible
– Ivaylo Strandjev
Jan 23 at 8:14
@lubgr in fact I am not certain what is the original intent of the OP, as the variable name is row I think storing the whole line is more plausible
– Ivaylo Strandjev
Jan 23 at 8:14
add a comment |
If you want to store the tokens of one line from std::cin
, separated by the standard mechanism as in the operator>>
overloads from <iostream>
(i.e., split by whitespace/newline), you can do it like this:
std::string line;
std::getline(std::cin, line);
std::stringstream ss{line};
const std::vector<std::string> tokens{std::istream_iterator<std::string>{ss},
std::istream_iterator<std::string>{}};
Note that this is not the most efficient solution, but it should work as expected: process only one line and use an existing mechanism to split this line into individual std::string
objects.
add a comment |
If you want to store the tokens of one line from std::cin
, separated by the standard mechanism as in the operator>>
overloads from <iostream>
(i.e., split by whitespace/newline), you can do it like this:
std::string line;
std::getline(std::cin, line);
std::stringstream ss{line};
const std::vector<std::string> tokens{std::istream_iterator<std::string>{ss},
std::istream_iterator<std::string>{}};
Note that this is not the most efficient solution, but it should work as expected: process only one line and use an existing mechanism to split this line into individual std::string
objects.
add a comment |
If you want to store the tokens of one line from std::cin
, separated by the standard mechanism as in the operator>>
overloads from <iostream>
(i.e., split by whitespace/newline), you can do it like this:
std::string line;
std::getline(std::cin, line);
std::stringstream ss{line};
const std::vector<std::string> tokens{std::istream_iterator<std::string>{ss},
std::istream_iterator<std::string>{}};
Note that this is not the most efficient solution, but it should work as expected: process only one line and use an existing mechanism to split this line into individual std::string
objects.
If you want to store the tokens of one line from std::cin
, separated by the standard mechanism as in the operator>>
overloads from <iostream>
(i.e., split by whitespace/newline), you can do it like this:
std::string line;
std::getline(std::cin, line);
std::stringstream ss{line};
const std::vector<std::string> tokens{std::istream_iterator<std::string>{ss},
std::istream_iterator<std::string>{}};
Note that this is not the most efficient solution, but it should work as expected: process only one line and use an existing mechanism to split this line into individual std::string
objects.
edited Jan 23 at 9:20
answered Jan 23 at 8:19


lubgrlubgr
11.9k21846
11.9k21846
add a comment |
add a comment |
You can't read newline by using the istream& operator >>
of string. This operator ignores whitespaces and will never return the string "n"
. Consider using getline instead.
getline()
won't return"n"
either.
– Sid S
Jan 23 at 8:26
1
No, it will not but it will serve the purpose to read a whole row.
– Ivaylo Strandjev
Jan 23 at 8:30
add a comment |
You can't read newline by using the istream& operator >>
of string. This operator ignores whitespaces and will never return the string "n"
. Consider using getline instead.
getline()
won't return"n"
either.
– Sid S
Jan 23 at 8:26
1
No, it will not but it will serve the purpose to read a whole row.
– Ivaylo Strandjev
Jan 23 at 8:30
add a comment |
You can't read newline by using the istream& operator >>
of string. This operator ignores whitespaces and will never return the string "n"
. Consider using getline instead.
You can't read newline by using the istream& operator >>
of string. This operator ignores whitespaces and will never return the string "n"
. Consider using getline instead.
answered Jan 23 at 8:03
Ivaylo StrandjevIvaylo Strandjev
56.5k1086142
56.5k1086142
getline()
won't return"n"
either.
– Sid S
Jan 23 at 8:26
1
No, it will not but it will serve the purpose to read a whole row.
– Ivaylo Strandjev
Jan 23 at 8:30
add a comment |
getline()
won't return"n"
either.
– Sid S
Jan 23 at 8:26
1
No, it will not but it will serve the purpose to read a whole row.
– Ivaylo Strandjev
Jan 23 at 8:30
getline()
won't return "n"
either.– Sid S
Jan 23 at 8:26
getline()
won't return "n"
either.– Sid S
Jan 23 at 8:26
1
1
No, it will not but it will serve the purpose to read a whole row.
– Ivaylo Strandjev
Jan 23 at 8:30
No, it will not but it will serve the purpose to read a whole row.
– Ivaylo Strandjev
Jan 23 at 8:30
add a comment |
lY,JyE Ceec6jg9glf7l0UCAwLOB,JO0XjV
8
cin >> row
discards whitespace, sorow
is never going to be"n"
. If you want to end on , break onrow == "\"
.– Sid S
Jan 23 at 7:55
3
Also consider breaking on
row.empty()
.– Sid S
Jan 23 at 8:02