Program that converts a number to a letter of the alphabet
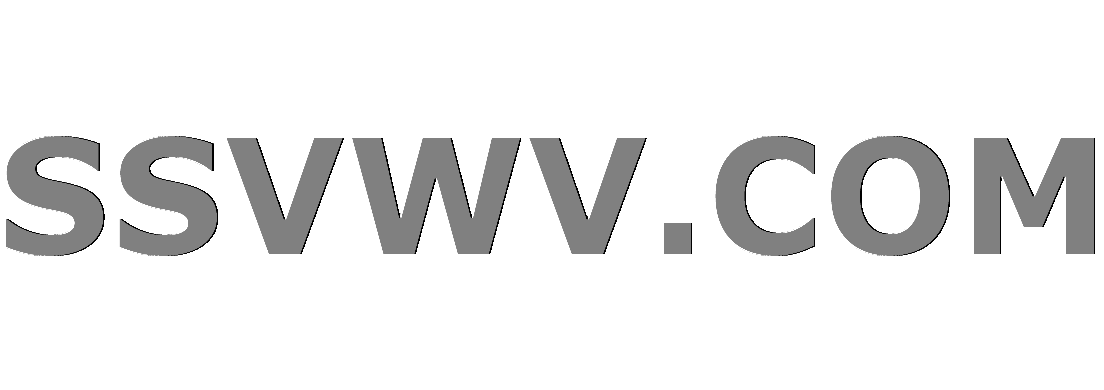
Multi tool use
$begingroup$
#include <iostream>
int main() {
int letter = 0;
std::cout << "Please type in a number: ";
while (1) {
std::cin >> letter;
//less than 1 or greater than 26
if (!(letter < 1 || 26 < letter)) {
std::cout << "The letter that corosponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
std::cout <<"The English Alphabet only has 26 letters. Try againn";
}
}
Gets a integer and prints it as a character. I had thought about using stdio instead of stream but I didn't want it to look scary to a beginner.
c++
$endgroup$
add a comment |
$begingroup$
#include <iostream>
int main() {
int letter = 0;
std::cout << "Please type in a number: ";
while (1) {
std::cin >> letter;
//less than 1 or greater than 26
if (!(letter < 1 || 26 < letter)) {
std::cout << "The letter that corosponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
std::cout <<"The English Alphabet only has 26 letters. Try againn";
}
}
Gets a integer and prints it as a character. I had thought about using stdio instead of stream but I didn't want it to look scary to a beginner.
c++
$endgroup$
add a comment |
$begingroup$
#include <iostream>
int main() {
int letter = 0;
std::cout << "Please type in a number: ";
while (1) {
std::cin >> letter;
//less than 1 or greater than 26
if (!(letter < 1 || 26 < letter)) {
std::cout << "The letter that corosponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
std::cout <<"The English Alphabet only has 26 letters. Try againn";
}
}
Gets a integer and prints it as a character. I had thought about using stdio instead of stream but I didn't want it to look scary to a beginner.
c++
$endgroup$
#include <iostream>
int main() {
int letter = 0;
std::cout << "Please type in a number: ";
while (1) {
std::cin >> letter;
//less than 1 or greater than 26
if (!(letter < 1 || 26 < letter)) {
std::cout << "The letter that corosponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
std::cout <<"The English Alphabet only has 26 letters. Try againn";
}
}
Gets a integer and prints it as a character. I had thought about using stdio instead of stream but I didn't want it to look scary to a beginner.
c++
c++
asked Mar 1 at 4:31


Alex AngelAlex Angel
613
613
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
$begingroup$
C++ does not mandate a particular character coding, so while your code is functionally correct on ASCII and ISO-8859 systems, it won't work in EBCDIC environments, for example. Even with the obvious fix to add 'A'-1
in place of the magic constant 64
, the program assumes that English letters have consecutive values, which just isn't the case in BCD encodings.
The portable approach is to index a string literal:
static const std::string_view alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
if (letter > 0 && letter <= alphabet.size()) {
std::cout << "Letter " << letter << " is '"
<< alphabet[letter-1]
<< "'n";
}
Other issues:
- Don't use
letter
before checking whetherstd::cin >> letter
succeeded. - Instead of an infinite loop, prefer a finite loop for reading inputs.
- Consider accepting input as command-line argument(s) as a more convenient alternative to using standard input.
- Spelling: "corresponds".
$endgroup$
5
$begingroup$
I'm always amazed what you can learn from an at first glance trivial task.
$endgroup$
– infinitezero
Mar 1 at 14:02
add a comment |
$begingroup$
The declaration int letter = 0;
is perhaps too early in the program. letter
is only used inside the while
loop, so it should be inside of the loop.
Initializing int letter = 0;
is misleading. The initial value of letter
is overwritten by the std::cin >> letter;
statement, so the initial value of 0
is never used, and could be removed.
while (1) {
should be written as while (true) {
. Both amount to an infinite loop, but the latter reads better.
26
is a magic number, so you might want to turn into a named constant. Still, it is a pretty obvious constant, so I’d actually be ok with leaving it as is. However ...
64
is a magic number which absolutely should be turned into a named constant ... or eliminated altogether. What does 64
represent? The ASCII character'@'
? Still not obvious what it is doing, or why. How about using the expression 'A' + (letter - 1)
? That is much clearer and better!
Problem: If the user enters the number "hello"
, the std:cin
stream will go into a fail
state, and executing std::cin >> letter;
subsequent times will continue to fail, and "The English Alphabet ... Try again"
will be repeated forever. You should check for the stream going into the fail
state, clear the error, then discard the invalid input, before returning to reading the next integer.
Outputting "The letter that coresponds [sic] to that value is"
inside the while
loop makes it look like you can enter several values and get the results in the loop, when in reality you can only get one translation before the program exits. You should loop for the user’s input, and break out of the loop once the input is valid, and print the translation outside, after the loop. Something like:
int letter;
do {
if (std::cin >> letter) {
if (letter >= 1 && letter <= 26)
break;
} else {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), 'n');
}
std::cout << "Try againn";
} while (true);
std::cout << "The letter that corresponds ...
$endgroup$
$begingroup$
You might want to check up on the correct usage of [sic]. In your answer you're using it to indicate that you changed the cited text to have correct spelling, while you should use [sic] to indicate that the original author made an error and you just cite as it was written. In case it's just autocorrect trolling you (and me), ignore what I just said. Or rather tell me and I'll delete my comment. ;)
$endgroup$
– Inarion
Mar 1 at 14:13
1
$begingroup$
@Inarion Yup! Autocorrect fixed what I had left unfixed, and clearly I blinked and didn’t notice. I’ve uncorrected it to the proper (intended) usage.
$endgroup$
– AJNeufeld
Mar 1 at 15:41
2
$begingroup$
Actually, @Ludisposed mistakenly corrected the spelling (presumably not seeing the[sic]
there).
$endgroup$
– Toby Speight
Mar 1 at 15:44
$begingroup$
Oops my bad! I am a simple man, I see red squiggle I do correct
$endgroup$
– Ludisposed
Mar 1 at 15:47
$begingroup$
"corresponds" has two r's, doesn't it?
$endgroup$
– Buttle Butkus
Mar 3 at 0:58
add a comment |
$begingroup$
I have five improvements to suggest. The first two are about sanitizing inputs, and are very important. The next two are about user interface design, and are minor. The last is about coding style, and is completely optional.
Fail elegantly on non-integer inputs. This can be done using the
fail()
,clear()
, andignore()
methods ofstd::cin
.Respond to
EOF
appropriately. This can be done usingstd::cin.eof()
.Repeat the prompt after printing an error message.
Check spelling of
"corresponds"
. Do not capitalize"alphabet"
In general, I dislike returning inside of a loop. For a clearer control flow, I would loop until getting a valid input, then, outside the loop convert to a letter.
#include <iostream>
#include <limits>
int main() {
int letter = 0;
while (1) {
std::cout << "Please type in a number: ";
std::cin >> letter;
if (std::cin.eof()) {
std::cout << std::endl;
return 1;
} else if (std::cin.fail()) {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(),'n');
std::cout <<"Integer input required. Try again.n";
} else if (letter < 1 || letter > 26) {
std::cout <<"The English alphabet only has 26 letters. Try again.n";
} else {
break;
}
}
std::cout << "The letter that corresponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f214512%2fprogram-that-converts-a-number-to-a-letter-of-the-alphabet%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
C++ does not mandate a particular character coding, so while your code is functionally correct on ASCII and ISO-8859 systems, it won't work in EBCDIC environments, for example. Even with the obvious fix to add 'A'-1
in place of the magic constant 64
, the program assumes that English letters have consecutive values, which just isn't the case in BCD encodings.
The portable approach is to index a string literal:
static const std::string_view alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
if (letter > 0 && letter <= alphabet.size()) {
std::cout << "Letter " << letter << " is '"
<< alphabet[letter-1]
<< "'n";
}
Other issues:
- Don't use
letter
before checking whetherstd::cin >> letter
succeeded. - Instead of an infinite loop, prefer a finite loop for reading inputs.
- Consider accepting input as command-line argument(s) as a more convenient alternative to using standard input.
- Spelling: "corresponds".
$endgroup$
5
$begingroup$
I'm always amazed what you can learn from an at first glance trivial task.
$endgroup$
– infinitezero
Mar 1 at 14:02
add a comment |
$begingroup$
C++ does not mandate a particular character coding, so while your code is functionally correct on ASCII and ISO-8859 systems, it won't work in EBCDIC environments, for example. Even with the obvious fix to add 'A'-1
in place of the magic constant 64
, the program assumes that English letters have consecutive values, which just isn't the case in BCD encodings.
The portable approach is to index a string literal:
static const std::string_view alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
if (letter > 0 && letter <= alphabet.size()) {
std::cout << "Letter " << letter << " is '"
<< alphabet[letter-1]
<< "'n";
}
Other issues:
- Don't use
letter
before checking whetherstd::cin >> letter
succeeded. - Instead of an infinite loop, prefer a finite loop for reading inputs.
- Consider accepting input as command-line argument(s) as a more convenient alternative to using standard input.
- Spelling: "corresponds".
$endgroup$
5
$begingroup$
I'm always amazed what you can learn from an at first glance trivial task.
$endgroup$
– infinitezero
Mar 1 at 14:02
add a comment |
$begingroup$
C++ does not mandate a particular character coding, so while your code is functionally correct on ASCII and ISO-8859 systems, it won't work in EBCDIC environments, for example. Even with the obvious fix to add 'A'-1
in place of the magic constant 64
, the program assumes that English letters have consecutive values, which just isn't the case in BCD encodings.
The portable approach is to index a string literal:
static const std::string_view alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
if (letter > 0 && letter <= alphabet.size()) {
std::cout << "Letter " << letter << " is '"
<< alphabet[letter-1]
<< "'n";
}
Other issues:
- Don't use
letter
before checking whetherstd::cin >> letter
succeeded. - Instead of an infinite loop, prefer a finite loop for reading inputs.
- Consider accepting input as command-line argument(s) as a more convenient alternative to using standard input.
- Spelling: "corresponds".
$endgroup$
C++ does not mandate a particular character coding, so while your code is functionally correct on ASCII and ISO-8859 systems, it won't work in EBCDIC environments, for example. Even with the obvious fix to add 'A'-1
in place of the magic constant 64
, the program assumes that English letters have consecutive values, which just isn't the case in BCD encodings.
The portable approach is to index a string literal:
static const std::string_view alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
if (letter > 0 && letter <= alphabet.size()) {
std::cout << "Letter " << letter << " is '"
<< alphabet[letter-1]
<< "'n";
}
Other issues:
- Don't use
letter
before checking whetherstd::cin >> letter
succeeded. - Instead of an infinite loop, prefer a finite loop for reading inputs.
- Consider accepting input as command-line argument(s) as a more convenient alternative to using standard input.
- Spelling: "corresponds".
answered Mar 1 at 8:06


Toby SpeightToby Speight
27k742118
27k742118
5
$begingroup$
I'm always amazed what you can learn from an at first glance trivial task.
$endgroup$
– infinitezero
Mar 1 at 14:02
add a comment |
5
$begingroup$
I'm always amazed what you can learn from an at first glance trivial task.
$endgroup$
– infinitezero
Mar 1 at 14:02
5
5
$begingroup$
I'm always amazed what you can learn from an at first glance trivial task.
$endgroup$
– infinitezero
Mar 1 at 14:02
$begingroup$
I'm always amazed what you can learn from an at first glance trivial task.
$endgroup$
– infinitezero
Mar 1 at 14:02
add a comment |
$begingroup$
The declaration int letter = 0;
is perhaps too early in the program. letter
is only used inside the while
loop, so it should be inside of the loop.
Initializing int letter = 0;
is misleading. The initial value of letter
is overwritten by the std::cin >> letter;
statement, so the initial value of 0
is never used, and could be removed.
while (1) {
should be written as while (true) {
. Both amount to an infinite loop, but the latter reads better.
26
is a magic number, so you might want to turn into a named constant. Still, it is a pretty obvious constant, so I’d actually be ok with leaving it as is. However ...
64
is a magic number which absolutely should be turned into a named constant ... or eliminated altogether. What does 64
represent? The ASCII character'@'
? Still not obvious what it is doing, or why. How about using the expression 'A' + (letter - 1)
? That is much clearer and better!
Problem: If the user enters the number "hello"
, the std:cin
stream will go into a fail
state, and executing std::cin >> letter;
subsequent times will continue to fail, and "The English Alphabet ... Try again"
will be repeated forever. You should check for the stream going into the fail
state, clear the error, then discard the invalid input, before returning to reading the next integer.
Outputting "The letter that coresponds [sic] to that value is"
inside the while
loop makes it look like you can enter several values and get the results in the loop, when in reality you can only get one translation before the program exits. You should loop for the user’s input, and break out of the loop once the input is valid, and print the translation outside, after the loop. Something like:
int letter;
do {
if (std::cin >> letter) {
if (letter >= 1 && letter <= 26)
break;
} else {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), 'n');
}
std::cout << "Try againn";
} while (true);
std::cout << "The letter that corresponds ...
$endgroup$
$begingroup$
You might want to check up on the correct usage of [sic]. In your answer you're using it to indicate that you changed the cited text to have correct spelling, while you should use [sic] to indicate that the original author made an error and you just cite as it was written. In case it's just autocorrect trolling you (and me), ignore what I just said. Or rather tell me and I'll delete my comment. ;)
$endgroup$
– Inarion
Mar 1 at 14:13
1
$begingroup$
@Inarion Yup! Autocorrect fixed what I had left unfixed, and clearly I blinked and didn’t notice. I’ve uncorrected it to the proper (intended) usage.
$endgroup$
– AJNeufeld
Mar 1 at 15:41
2
$begingroup$
Actually, @Ludisposed mistakenly corrected the spelling (presumably not seeing the[sic]
there).
$endgroup$
– Toby Speight
Mar 1 at 15:44
$begingroup$
Oops my bad! I am a simple man, I see red squiggle I do correct
$endgroup$
– Ludisposed
Mar 1 at 15:47
$begingroup$
"corresponds" has two r's, doesn't it?
$endgroup$
– Buttle Butkus
Mar 3 at 0:58
add a comment |
$begingroup$
The declaration int letter = 0;
is perhaps too early in the program. letter
is only used inside the while
loop, so it should be inside of the loop.
Initializing int letter = 0;
is misleading. The initial value of letter
is overwritten by the std::cin >> letter;
statement, so the initial value of 0
is never used, and could be removed.
while (1) {
should be written as while (true) {
. Both amount to an infinite loop, but the latter reads better.
26
is a magic number, so you might want to turn into a named constant. Still, it is a pretty obvious constant, so I’d actually be ok with leaving it as is. However ...
64
is a magic number which absolutely should be turned into a named constant ... or eliminated altogether. What does 64
represent? The ASCII character'@'
? Still not obvious what it is doing, or why. How about using the expression 'A' + (letter - 1)
? That is much clearer and better!
Problem: If the user enters the number "hello"
, the std:cin
stream will go into a fail
state, and executing std::cin >> letter;
subsequent times will continue to fail, and "The English Alphabet ... Try again"
will be repeated forever. You should check for the stream going into the fail
state, clear the error, then discard the invalid input, before returning to reading the next integer.
Outputting "The letter that coresponds [sic] to that value is"
inside the while
loop makes it look like you can enter several values and get the results in the loop, when in reality you can only get one translation before the program exits. You should loop for the user’s input, and break out of the loop once the input is valid, and print the translation outside, after the loop. Something like:
int letter;
do {
if (std::cin >> letter) {
if (letter >= 1 && letter <= 26)
break;
} else {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), 'n');
}
std::cout << "Try againn";
} while (true);
std::cout << "The letter that corresponds ...
$endgroup$
$begingroup$
You might want to check up on the correct usage of [sic]. In your answer you're using it to indicate that you changed the cited text to have correct spelling, while you should use [sic] to indicate that the original author made an error and you just cite as it was written. In case it's just autocorrect trolling you (and me), ignore what I just said. Or rather tell me and I'll delete my comment. ;)
$endgroup$
– Inarion
Mar 1 at 14:13
1
$begingroup$
@Inarion Yup! Autocorrect fixed what I had left unfixed, and clearly I blinked and didn’t notice. I’ve uncorrected it to the proper (intended) usage.
$endgroup$
– AJNeufeld
Mar 1 at 15:41
2
$begingroup$
Actually, @Ludisposed mistakenly corrected the spelling (presumably not seeing the[sic]
there).
$endgroup$
– Toby Speight
Mar 1 at 15:44
$begingroup$
Oops my bad! I am a simple man, I see red squiggle I do correct
$endgroup$
– Ludisposed
Mar 1 at 15:47
$begingroup$
"corresponds" has two r's, doesn't it?
$endgroup$
– Buttle Butkus
Mar 3 at 0:58
add a comment |
$begingroup$
The declaration int letter = 0;
is perhaps too early in the program. letter
is only used inside the while
loop, so it should be inside of the loop.
Initializing int letter = 0;
is misleading. The initial value of letter
is overwritten by the std::cin >> letter;
statement, so the initial value of 0
is never used, and could be removed.
while (1) {
should be written as while (true) {
. Both amount to an infinite loop, but the latter reads better.
26
is a magic number, so you might want to turn into a named constant. Still, it is a pretty obvious constant, so I’d actually be ok with leaving it as is. However ...
64
is a magic number which absolutely should be turned into a named constant ... or eliminated altogether. What does 64
represent? The ASCII character'@'
? Still not obvious what it is doing, or why. How about using the expression 'A' + (letter - 1)
? That is much clearer and better!
Problem: If the user enters the number "hello"
, the std:cin
stream will go into a fail
state, and executing std::cin >> letter;
subsequent times will continue to fail, and "The English Alphabet ... Try again"
will be repeated forever. You should check for the stream going into the fail
state, clear the error, then discard the invalid input, before returning to reading the next integer.
Outputting "The letter that coresponds [sic] to that value is"
inside the while
loop makes it look like you can enter several values and get the results in the loop, when in reality you can only get one translation before the program exits. You should loop for the user’s input, and break out of the loop once the input is valid, and print the translation outside, after the loop. Something like:
int letter;
do {
if (std::cin >> letter) {
if (letter >= 1 && letter <= 26)
break;
} else {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), 'n');
}
std::cout << "Try againn";
} while (true);
std::cout << "The letter that corresponds ...
$endgroup$
The declaration int letter = 0;
is perhaps too early in the program. letter
is only used inside the while
loop, so it should be inside of the loop.
Initializing int letter = 0;
is misleading. The initial value of letter
is overwritten by the std::cin >> letter;
statement, so the initial value of 0
is never used, and could be removed.
while (1) {
should be written as while (true) {
. Both amount to an infinite loop, but the latter reads better.
26
is a magic number, so you might want to turn into a named constant. Still, it is a pretty obvious constant, so I’d actually be ok with leaving it as is. However ...
64
is a magic number which absolutely should be turned into a named constant ... or eliminated altogether. What does 64
represent? The ASCII character'@'
? Still not obvious what it is doing, or why. How about using the expression 'A' + (letter - 1)
? That is much clearer and better!
Problem: If the user enters the number "hello"
, the std:cin
stream will go into a fail
state, and executing std::cin >> letter;
subsequent times will continue to fail, and "The English Alphabet ... Try again"
will be repeated forever. You should check for the stream going into the fail
state, clear the error, then discard the invalid input, before returning to reading the next integer.
Outputting "The letter that coresponds [sic] to that value is"
inside the while
loop makes it look like you can enter several values and get the results in the loop, when in reality you can only get one translation before the program exits. You should loop for the user’s input, and break out of the loop once the input is valid, and print the translation outside, after the loop. Something like:
int letter;
do {
if (std::cin >> letter) {
if (letter >= 1 && letter <= 26)
break;
} else {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), 'n');
}
std::cout << "Try againn";
} while (true);
std::cout << "The letter that corresponds ...
edited Mar 1 at 15:38
answered Mar 1 at 6:30
AJNeufeldAJNeufeld
6,5891621
6,5891621
$begingroup$
You might want to check up on the correct usage of [sic]. In your answer you're using it to indicate that you changed the cited text to have correct spelling, while you should use [sic] to indicate that the original author made an error and you just cite as it was written. In case it's just autocorrect trolling you (and me), ignore what I just said. Or rather tell me and I'll delete my comment. ;)
$endgroup$
– Inarion
Mar 1 at 14:13
1
$begingroup$
@Inarion Yup! Autocorrect fixed what I had left unfixed, and clearly I blinked and didn’t notice. I’ve uncorrected it to the proper (intended) usage.
$endgroup$
– AJNeufeld
Mar 1 at 15:41
2
$begingroup$
Actually, @Ludisposed mistakenly corrected the spelling (presumably not seeing the[sic]
there).
$endgroup$
– Toby Speight
Mar 1 at 15:44
$begingroup$
Oops my bad! I am a simple man, I see red squiggle I do correct
$endgroup$
– Ludisposed
Mar 1 at 15:47
$begingroup$
"corresponds" has two r's, doesn't it?
$endgroup$
– Buttle Butkus
Mar 3 at 0:58
add a comment |
$begingroup$
You might want to check up on the correct usage of [sic]. In your answer you're using it to indicate that you changed the cited text to have correct spelling, while you should use [sic] to indicate that the original author made an error and you just cite as it was written. In case it's just autocorrect trolling you (and me), ignore what I just said. Or rather tell me and I'll delete my comment. ;)
$endgroup$
– Inarion
Mar 1 at 14:13
1
$begingroup$
@Inarion Yup! Autocorrect fixed what I had left unfixed, and clearly I blinked and didn’t notice. I’ve uncorrected it to the proper (intended) usage.
$endgroup$
– AJNeufeld
Mar 1 at 15:41
2
$begingroup$
Actually, @Ludisposed mistakenly corrected the spelling (presumably not seeing the[sic]
there).
$endgroup$
– Toby Speight
Mar 1 at 15:44
$begingroup$
Oops my bad! I am a simple man, I see red squiggle I do correct
$endgroup$
– Ludisposed
Mar 1 at 15:47
$begingroup$
"corresponds" has two r's, doesn't it?
$endgroup$
– Buttle Butkus
Mar 3 at 0:58
$begingroup$
You might want to check up on the correct usage of [sic]. In your answer you're using it to indicate that you changed the cited text to have correct spelling, while you should use [sic] to indicate that the original author made an error and you just cite as it was written. In case it's just autocorrect trolling you (and me), ignore what I just said. Or rather tell me and I'll delete my comment. ;)
$endgroup$
– Inarion
Mar 1 at 14:13
$begingroup$
You might want to check up on the correct usage of [sic]. In your answer you're using it to indicate that you changed the cited text to have correct spelling, while you should use [sic] to indicate that the original author made an error and you just cite as it was written. In case it's just autocorrect trolling you (and me), ignore what I just said. Or rather tell me and I'll delete my comment. ;)
$endgroup$
– Inarion
Mar 1 at 14:13
1
1
$begingroup$
@Inarion Yup! Autocorrect fixed what I had left unfixed, and clearly I blinked and didn’t notice. I’ve uncorrected it to the proper (intended) usage.
$endgroup$
– AJNeufeld
Mar 1 at 15:41
$begingroup$
@Inarion Yup! Autocorrect fixed what I had left unfixed, and clearly I blinked and didn’t notice. I’ve uncorrected it to the proper (intended) usage.
$endgroup$
– AJNeufeld
Mar 1 at 15:41
2
2
$begingroup$
Actually, @Ludisposed mistakenly corrected the spelling (presumably not seeing the
[sic]
there).$endgroup$
– Toby Speight
Mar 1 at 15:44
$begingroup$
Actually, @Ludisposed mistakenly corrected the spelling (presumably not seeing the
[sic]
there).$endgroup$
– Toby Speight
Mar 1 at 15:44
$begingroup$
Oops my bad! I am a simple man, I see red squiggle I do correct
$endgroup$
– Ludisposed
Mar 1 at 15:47
$begingroup$
Oops my bad! I am a simple man, I see red squiggle I do correct
$endgroup$
– Ludisposed
Mar 1 at 15:47
$begingroup$
"corresponds" has two r's, doesn't it?
$endgroup$
– Buttle Butkus
Mar 3 at 0:58
$begingroup$
"corresponds" has two r's, doesn't it?
$endgroup$
– Buttle Butkus
Mar 3 at 0:58
add a comment |
$begingroup$
I have five improvements to suggest. The first two are about sanitizing inputs, and are very important. The next two are about user interface design, and are minor. The last is about coding style, and is completely optional.
Fail elegantly on non-integer inputs. This can be done using the
fail()
,clear()
, andignore()
methods ofstd::cin
.Respond to
EOF
appropriately. This can be done usingstd::cin.eof()
.Repeat the prompt after printing an error message.
Check spelling of
"corresponds"
. Do not capitalize"alphabet"
In general, I dislike returning inside of a loop. For a clearer control flow, I would loop until getting a valid input, then, outside the loop convert to a letter.
#include <iostream>
#include <limits>
int main() {
int letter = 0;
while (1) {
std::cout << "Please type in a number: ";
std::cin >> letter;
if (std::cin.eof()) {
std::cout << std::endl;
return 1;
} else if (std::cin.fail()) {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(),'n');
std::cout <<"Integer input required. Try again.n";
} else if (letter < 1 || letter > 26) {
std::cout <<"The English alphabet only has 26 letters. Try again.n";
} else {
break;
}
}
std::cout << "The letter that corresponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
$endgroup$
add a comment |
$begingroup$
I have five improvements to suggest. The first two are about sanitizing inputs, and are very important. The next two are about user interface design, and are minor. The last is about coding style, and is completely optional.
Fail elegantly on non-integer inputs. This can be done using the
fail()
,clear()
, andignore()
methods ofstd::cin
.Respond to
EOF
appropriately. This can be done usingstd::cin.eof()
.Repeat the prompt after printing an error message.
Check spelling of
"corresponds"
. Do not capitalize"alphabet"
In general, I dislike returning inside of a loop. For a clearer control flow, I would loop until getting a valid input, then, outside the loop convert to a letter.
#include <iostream>
#include <limits>
int main() {
int letter = 0;
while (1) {
std::cout << "Please type in a number: ";
std::cin >> letter;
if (std::cin.eof()) {
std::cout << std::endl;
return 1;
} else if (std::cin.fail()) {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(),'n');
std::cout <<"Integer input required. Try again.n";
} else if (letter < 1 || letter > 26) {
std::cout <<"The English alphabet only has 26 letters. Try again.n";
} else {
break;
}
}
std::cout << "The letter that corresponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
$endgroup$
add a comment |
$begingroup$
I have five improvements to suggest. The first two are about sanitizing inputs, and are very important. The next two are about user interface design, and are minor. The last is about coding style, and is completely optional.
Fail elegantly on non-integer inputs. This can be done using the
fail()
,clear()
, andignore()
methods ofstd::cin
.Respond to
EOF
appropriately. This can be done usingstd::cin.eof()
.Repeat the prompt after printing an error message.
Check spelling of
"corresponds"
. Do not capitalize"alphabet"
In general, I dislike returning inside of a loop. For a clearer control flow, I would loop until getting a valid input, then, outside the loop convert to a letter.
#include <iostream>
#include <limits>
int main() {
int letter = 0;
while (1) {
std::cout << "Please type in a number: ";
std::cin >> letter;
if (std::cin.eof()) {
std::cout << std::endl;
return 1;
} else if (std::cin.fail()) {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(),'n');
std::cout <<"Integer input required. Try again.n";
} else if (letter < 1 || letter > 26) {
std::cout <<"The English alphabet only has 26 letters. Try again.n";
} else {
break;
}
}
std::cout << "The letter that corresponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
$endgroup$
I have five improvements to suggest. The first two are about sanitizing inputs, and are very important. The next two are about user interface design, and are minor. The last is about coding style, and is completely optional.
Fail elegantly on non-integer inputs. This can be done using the
fail()
,clear()
, andignore()
methods ofstd::cin
.Respond to
EOF
appropriately. This can be done usingstd::cin.eof()
.Repeat the prompt after printing an error message.
Check spelling of
"corresponds"
. Do not capitalize"alphabet"
In general, I dislike returning inside of a loop. For a clearer control flow, I would loop until getting a valid input, then, outside the loop convert to a letter.
#include <iostream>
#include <limits>
int main() {
int letter = 0;
while (1) {
std::cout << "Please type in a number: ";
std::cin >> letter;
if (std::cin.eof()) {
std::cout << std::endl;
return 1;
} else if (std::cin.fail()) {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(),'n');
std::cout <<"Integer input required. Try again.n";
} else if (letter < 1 || letter > 26) {
std::cout <<"The English alphabet only has 26 letters. Try again.n";
} else {
break;
}
}
std::cout << "The letter that corresponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
answered Mar 1 at 6:33
Benjamin KuykendallBenjamin Kuykendall
72829
72829
add a comment |
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f214512%2fprogram-that-converts-a-number-to-a-letter-of-the-alphabet%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
i2oUB,cdBcYHl qfuIQGWD,TwyYOZl5dcH9C,4JSq2aFo aWjZQ3cDgQpoAhiPXI,lSW240eG3nJouAvJV2,W6OQDtrwk