XML node removal method with 5 arguments
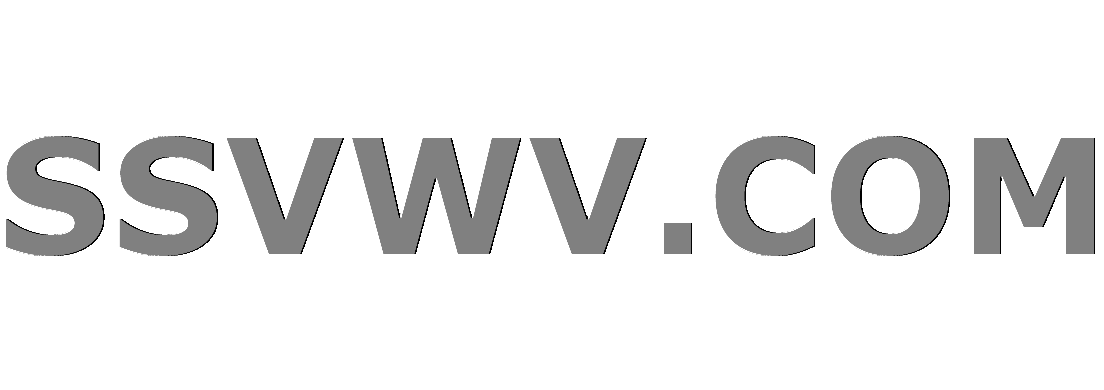
Multi tool use
$begingroup$
I read somewhere that you should try to have as few method arguments as possible to make code easier to read, understand and use. I agree with this to a point but I'm not sure how I can make the following method easier. I think all 5 of the arguments are required.
Should I leave it as is? I think, if I was to add some comments, I may remember what this does if I come back to it later but there's a chance I won't know exactly what each argument is for.
public static void RemoveXMLNode(string pathToDocument, string descendant, string element, string elementValue,string newDocumentPath)
{
var xDoc = XDocument.Load(pathToDocument);
xDoc.Descendants(descendant)
.Where(n => (string)n.Element(element) == elementValue)
.Remove();
xDoc.Save(newDocumentPath);
}
c# beginner
$endgroup$
add a comment |
$begingroup$
I read somewhere that you should try to have as few method arguments as possible to make code easier to read, understand and use. I agree with this to a point but I'm not sure how I can make the following method easier. I think all 5 of the arguments are required.
Should I leave it as is? I think, if I was to add some comments, I may remember what this does if I come back to it later but there's a chance I won't know exactly what each argument is for.
public static void RemoveXMLNode(string pathToDocument, string descendant, string element, string elementValue,string newDocumentPath)
{
var xDoc = XDocument.Load(pathToDocument);
xDoc.Descendants(descendant)
.Where(n => (string)n.Element(element) == elementValue)
.Remove();
xDoc.Save(newDocumentPath);
}
c# beginner
$endgroup$
add a comment |
$begingroup$
I read somewhere that you should try to have as few method arguments as possible to make code easier to read, understand and use. I agree with this to a point but I'm not sure how I can make the following method easier. I think all 5 of the arguments are required.
Should I leave it as is? I think, if I was to add some comments, I may remember what this does if I come back to it later but there's a chance I won't know exactly what each argument is for.
public static void RemoveXMLNode(string pathToDocument, string descendant, string element, string elementValue,string newDocumentPath)
{
var xDoc = XDocument.Load(pathToDocument);
xDoc.Descendants(descendant)
.Where(n => (string)n.Element(element) == elementValue)
.Remove();
xDoc.Save(newDocumentPath);
}
c# beginner
$endgroup$
I read somewhere that you should try to have as few method arguments as possible to make code easier to read, understand and use. I agree with this to a point but I'm not sure how I can make the following method easier. I think all 5 of the arguments are required.
Should I leave it as is? I think, if I was to add some comments, I may remember what this does if I come back to it later but there's a chance I won't know exactly what each argument is for.
public static void RemoveXMLNode(string pathToDocument, string descendant, string element, string elementValue,string newDocumentPath)
{
var xDoc = XDocument.Load(pathToDocument);
xDoc.Descendants(descendant)
.Where(n => (string)n.Element(element) == elementValue)
.Remove();
xDoc.Save(newDocumentPath);
}
c# beginner
c# beginner
edited Feb 15 at 11:33


t3chb0t
35k752124
35k752124
asked Feb 15 at 11:06
Syntax ErrorSyntax Error
20215
20215
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
$begingroup$
The method has definitely too many arguments and the reason for that is because it does too much. When you look at its name you could think it removes a XML node but under the cover it does three things:
- it loads a document
- it then does its job of removing a node
- it then saves the document under new name
If you properly separated these three concerns your APIs would have much simpler signatures.
$endgroup$
$begingroup$
Ok I have edited my post to try and refactor. I think it is an improvement but I still seem to need 4 arguments on my node removal part.
$endgroup$
– Syntax Error
Feb 15 at 11:32
$begingroup$
@SyntaxError you're on the right track ;-) but unfortunatelly it's not allowed to add the improved version to the question. You can post a self-answer or a follow-up if you want us to take another look at your new code ;-] I had to rollback your edit.
$endgroup$
– t3chb0t
Feb 15 at 11:34
$begingroup$
Oh ok i didn't know that! Posted an answer instead. Thanks :)
$endgroup$
– Syntax Error
Feb 15 at 11:40
add a comment |
$begingroup$
As a follow-up to t3chb0t's and your self-answer, I agree that whilst the original RemoveXMLNode
method you have written in your question is technically a one-liner, it is doing a lot.
In response to your self-answer, I have a few pointer:
- In your case, I don't think you need a separate
LoadXMLDocument
method. The lineXDocument.load(path)
is easy enough to understand. - Regarding you new
RemoveXMLNode
method, you have two options:
1. Pass by Reference
In your new method, you are requesting an XDocument
argument. Whilst XDocument
is a reference type and can be modified through its public methods, you may benefit from using the ref
keyword so that you pass the whole object as a reference. This means that any changes to the object you are passing happen on the original object. So to apply this, you would simply change the method arguments to:
// VVV - Note the 'ref' keyword!
private static void RemoveXMLNode(ref XDocument doc, string descendant, string element, string elementValue)
You would then use the method like so:
// VVV - Note the 'ref' keyword!
RemoveXMLNode(ref doc, "Questions", "quPage", "PAGE60");
You can find more information on the difference between Pass By Reference and Pass By Copy over here.
There is also some more information on passing Reference Type classes from Jon Skeet's article over here.
I also wrote some dummy code to try out and demonstrate this behaviour over here.
2. Extension Method
This simply creates an extension method for your XDocument
object. You do so by telling the compiler which of your arguments' object you are extending using the this
keyword. When applying, you will actually reduce the number of required parameters by 1, effectively making it shorter.
The only requirement for this option is that your extension method(s) must be in a non-generic and non-nested static class.
You would write your method like so:
// VVVV - Note the 'this' keyword!
private static void RemoveXMLNode(this XDocument doc, string descendant, string element, string elementValue)
Now, you would call this method like so:
// Only three arguments!
doc.RemoveXMLNode("Questions", "quPage", "PAGE60");
$endgroup$
4
$begingroup$
Regarding #1, you're wrong: becauseXDocument
is a reference type, the only thing that gets copied is a reference to theXDocument
instance, not the instance itself.
$endgroup$
– Pieter Witvoet
Feb 15 at 12:36
$begingroup$
@PieterWitvoet If you're referring to the fact that I mentioned that it's passing a copy, then (on further digging around) I agree and I will modify my answer. If you're referring to the use ofref
, I don't agree, as it still works.
$endgroup$
– Tom
Feb 15 at 12:57
1
$begingroup$
Addingref
won't break the code, but you'll be passing a reference to a reference around for no good reason. It'll clutter the code, send a confusing signal to other programmers and has a (tiny) performance implication. All for no benefit.
$endgroup$
– Pieter Witvoet
Feb 15 at 13:05
add a comment |
$begingroup$
I went with the extension method in the end as it produced the least code
Extension method
public static class XDocumentExtensions
{
public static void RemoveXMLNode(this XDocument doc, string descendant, string element, string elementValue)
{
doc.Descendants(descendant)
.Where(n => (string)n.Element(element) == elementValue)
.Remove();
}
}
Main program
class Program
{
static void Main(string args)
{
XDocument doc = XDocument.Load(@"C:TempOriginal.xml");
doc.RemoveXMLNode("Questions", "quPage", "PAGE60");
doc.Save(@"C:TempNewXmlDoc.xml");
}
}
$endgroup$
$begingroup$
I would add theref
keyword to theXDocument
argument in theRemoveXMLNode
method, so that you actually pass by reference. As it is, it will create a copy ofdoc
and remove the node from the copy. The original will stay with the node you are trying to remove.
$endgroup$
– Tom
Feb 15 at 11:51
5
$begingroup$
@Tom:XDocument
is a class and thus a reference type. What you are saying only applies to value types.
$endgroup$
– Pieter Witvoet
Feb 15 at 12:24
$begingroup$
While OPs are encouraged to answer their own questions bear in mind that "Every answer must make at least one insightful observation about the code in the question. Answers that merely provide an alternate solution with no explanation or justification do not constitute valid Code Review answers and may be deleted."...
$endgroup$
– Sᴀᴍ Onᴇᴌᴀ
Feb 15 at 18:10
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f213509%2fxml-node-removal-method-with-5-arguments%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
The method has definitely too many arguments and the reason for that is because it does too much. When you look at its name you could think it removes a XML node but under the cover it does three things:
- it loads a document
- it then does its job of removing a node
- it then saves the document under new name
If you properly separated these three concerns your APIs would have much simpler signatures.
$endgroup$
$begingroup$
Ok I have edited my post to try and refactor. I think it is an improvement but I still seem to need 4 arguments on my node removal part.
$endgroup$
– Syntax Error
Feb 15 at 11:32
$begingroup$
@SyntaxError you're on the right track ;-) but unfortunatelly it's not allowed to add the improved version to the question. You can post a self-answer or a follow-up if you want us to take another look at your new code ;-] I had to rollback your edit.
$endgroup$
– t3chb0t
Feb 15 at 11:34
$begingroup$
Oh ok i didn't know that! Posted an answer instead. Thanks :)
$endgroup$
– Syntax Error
Feb 15 at 11:40
add a comment |
$begingroup$
The method has definitely too many arguments and the reason for that is because it does too much. When you look at its name you could think it removes a XML node but under the cover it does three things:
- it loads a document
- it then does its job of removing a node
- it then saves the document under new name
If you properly separated these three concerns your APIs would have much simpler signatures.
$endgroup$
$begingroup$
Ok I have edited my post to try and refactor. I think it is an improvement but I still seem to need 4 arguments on my node removal part.
$endgroup$
– Syntax Error
Feb 15 at 11:32
$begingroup$
@SyntaxError you're on the right track ;-) but unfortunatelly it's not allowed to add the improved version to the question. You can post a self-answer or a follow-up if you want us to take another look at your new code ;-] I had to rollback your edit.
$endgroup$
– t3chb0t
Feb 15 at 11:34
$begingroup$
Oh ok i didn't know that! Posted an answer instead. Thanks :)
$endgroup$
– Syntax Error
Feb 15 at 11:40
add a comment |
$begingroup$
The method has definitely too many arguments and the reason for that is because it does too much. When you look at its name you could think it removes a XML node but under the cover it does three things:
- it loads a document
- it then does its job of removing a node
- it then saves the document under new name
If you properly separated these three concerns your APIs would have much simpler signatures.
$endgroup$
The method has definitely too many arguments and the reason for that is because it does too much. When you look at its name you could think it removes a XML node but under the cover it does three things:
- it loads a document
- it then does its job of removing a node
- it then saves the document under new name
If you properly separated these three concerns your APIs would have much simpler signatures.
answered Feb 15 at 11:24


t3chb0tt3chb0t
35k752124
35k752124
$begingroup$
Ok I have edited my post to try and refactor. I think it is an improvement but I still seem to need 4 arguments on my node removal part.
$endgroup$
– Syntax Error
Feb 15 at 11:32
$begingroup$
@SyntaxError you're on the right track ;-) but unfortunatelly it's not allowed to add the improved version to the question. You can post a self-answer or a follow-up if you want us to take another look at your new code ;-] I had to rollback your edit.
$endgroup$
– t3chb0t
Feb 15 at 11:34
$begingroup$
Oh ok i didn't know that! Posted an answer instead. Thanks :)
$endgroup$
– Syntax Error
Feb 15 at 11:40
add a comment |
$begingroup$
Ok I have edited my post to try and refactor. I think it is an improvement but I still seem to need 4 arguments on my node removal part.
$endgroup$
– Syntax Error
Feb 15 at 11:32
$begingroup$
@SyntaxError you're on the right track ;-) but unfortunatelly it's not allowed to add the improved version to the question. You can post a self-answer or a follow-up if you want us to take another look at your new code ;-] I had to rollback your edit.
$endgroup$
– t3chb0t
Feb 15 at 11:34
$begingroup$
Oh ok i didn't know that! Posted an answer instead. Thanks :)
$endgroup$
– Syntax Error
Feb 15 at 11:40
$begingroup$
Ok I have edited my post to try and refactor. I think it is an improvement but I still seem to need 4 arguments on my node removal part.
$endgroup$
– Syntax Error
Feb 15 at 11:32
$begingroup$
Ok I have edited my post to try and refactor. I think it is an improvement but I still seem to need 4 arguments on my node removal part.
$endgroup$
– Syntax Error
Feb 15 at 11:32
$begingroup$
@SyntaxError you're on the right track ;-) but unfortunatelly it's not allowed to add the improved version to the question. You can post a self-answer or a follow-up if you want us to take another look at your new code ;-] I had to rollback your edit.
$endgroup$
– t3chb0t
Feb 15 at 11:34
$begingroup$
@SyntaxError you're on the right track ;-) but unfortunatelly it's not allowed to add the improved version to the question. You can post a self-answer or a follow-up if you want us to take another look at your new code ;-] I had to rollback your edit.
$endgroup$
– t3chb0t
Feb 15 at 11:34
$begingroup$
Oh ok i didn't know that! Posted an answer instead. Thanks :)
$endgroup$
– Syntax Error
Feb 15 at 11:40
$begingroup$
Oh ok i didn't know that! Posted an answer instead. Thanks :)
$endgroup$
– Syntax Error
Feb 15 at 11:40
add a comment |
$begingroup$
As a follow-up to t3chb0t's and your self-answer, I agree that whilst the original RemoveXMLNode
method you have written in your question is technically a one-liner, it is doing a lot.
In response to your self-answer, I have a few pointer:
- In your case, I don't think you need a separate
LoadXMLDocument
method. The lineXDocument.load(path)
is easy enough to understand. - Regarding you new
RemoveXMLNode
method, you have two options:
1. Pass by Reference
In your new method, you are requesting an XDocument
argument. Whilst XDocument
is a reference type and can be modified through its public methods, you may benefit from using the ref
keyword so that you pass the whole object as a reference. This means that any changes to the object you are passing happen on the original object. So to apply this, you would simply change the method arguments to:
// VVV - Note the 'ref' keyword!
private static void RemoveXMLNode(ref XDocument doc, string descendant, string element, string elementValue)
You would then use the method like so:
// VVV - Note the 'ref' keyword!
RemoveXMLNode(ref doc, "Questions", "quPage", "PAGE60");
You can find more information on the difference between Pass By Reference and Pass By Copy over here.
There is also some more information on passing Reference Type classes from Jon Skeet's article over here.
I also wrote some dummy code to try out and demonstrate this behaviour over here.
2. Extension Method
This simply creates an extension method for your XDocument
object. You do so by telling the compiler which of your arguments' object you are extending using the this
keyword. When applying, you will actually reduce the number of required parameters by 1, effectively making it shorter.
The only requirement for this option is that your extension method(s) must be in a non-generic and non-nested static class.
You would write your method like so:
// VVVV - Note the 'this' keyword!
private static void RemoveXMLNode(this XDocument doc, string descendant, string element, string elementValue)
Now, you would call this method like so:
// Only three arguments!
doc.RemoveXMLNode("Questions", "quPage", "PAGE60");
$endgroup$
4
$begingroup$
Regarding #1, you're wrong: becauseXDocument
is a reference type, the only thing that gets copied is a reference to theXDocument
instance, not the instance itself.
$endgroup$
– Pieter Witvoet
Feb 15 at 12:36
$begingroup$
@PieterWitvoet If you're referring to the fact that I mentioned that it's passing a copy, then (on further digging around) I agree and I will modify my answer. If you're referring to the use ofref
, I don't agree, as it still works.
$endgroup$
– Tom
Feb 15 at 12:57
1
$begingroup$
Addingref
won't break the code, but you'll be passing a reference to a reference around for no good reason. It'll clutter the code, send a confusing signal to other programmers and has a (tiny) performance implication. All for no benefit.
$endgroup$
– Pieter Witvoet
Feb 15 at 13:05
add a comment |
$begingroup$
As a follow-up to t3chb0t's and your self-answer, I agree that whilst the original RemoveXMLNode
method you have written in your question is technically a one-liner, it is doing a lot.
In response to your self-answer, I have a few pointer:
- In your case, I don't think you need a separate
LoadXMLDocument
method. The lineXDocument.load(path)
is easy enough to understand. - Regarding you new
RemoveXMLNode
method, you have two options:
1. Pass by Reference
In your new method, you are requesting an XDocument
argument. Whilst XDocument
is a reference type and can be modified through its public methods, you may benefit from using the ref
keyword so that you pass the whole object as a reference. This means that any changes to the object you are passing happen on the original object. So to apply this, you would simply change the method arguments to:
// VVV - Note the 'ref' keyword!
private static void RemoveXMLNode(ref XDocument doc, string descendant, string element, string elementValue)
You would then use the method like so:
// VVV - Note the 'ref' keyword!
RemoveXMLNode(ref doc, "Questions", "quPage", "PAGE60");
You can find more information on the difference between Pass By Reference and Pass By Copy over here.
There is also some more information on passing Reference Type classes from Jon Skeet's article over here.
I also wrote some dummy code to try out and demonstrate this behaviour over here.
2. Extension Method
This simply creates an extension method for your XDocument
object. You do so by telling the compiler which of your arguments' object you are extending using the this
keyword. When applying, you will actually reduce the number of required parameters by 1, effectively making it shorter.
The only requirement for this option is that your extension method(s) must be in a non-generic and non-nested static class.
You would write your method like so:
// VVVV - Note the 'this' keyword!
private static void RemoveXMLNode(this XDocument doc, string descendant, string element, string elementValue)
Now, you would call this method like so:
// Only three arguments!
doc.RemoveXMLNode("Questions", "quPage", "PAGE60");
$endgroup$
4
$begingroup$
Regarding #1, you're wrong: becauseXDocument
is a reference type, the only thing that gets copied is a reference to theXDocument
instance, not the instance itself.
$endgroup$
– Pieter Witvoet
Feb 15 at 12:36
$begingroup$
@PieterWitvoet If you're referring to the fact that I mentioned that it's passing a copy, then (on further digging around) I agree and I will modify my answer. If you're referring to the use ofref
, I don't agree, as it still works.
$endgroup$
– Tom
Feb 15 at 12:57
1
$begingroup$
Addingref
won't break the code, but you'll be passing a reference to a reference around for no good reason. It'll clutter the code, send a confusing signal to other programmers and has a (tiny) performance implication. All for no benefit.
$endgroup$
– Pieter Witvoet
Feb 15 at 13:05
add a comment |
$begingroup$
As a follow-up to t3chb0t's and your self-answer, I agree that whilst the original RemoveXMLNode
method you have written in your question is technically a one-liner, it is doing a lot.
In response to your self-answer, I have a few pointer:
- In your case, I don't think you need a separate
LoadXMLDocument
method. The lineXDocument.load(path)
is easy enough to understand. - Regarding you new
RemoveXMLNode
method, you have two options:
1. Pass by Reference
In your new method, you are requesting an XDocument
argument. Whilst XDocument
is a reference type and can be modified through its public methods, you may benefit from using the ref
keyword so that you pass the whole object as a reference. This means that any changes to the object you are passing happen on the original object. So to apply this, you would simply change the method arguments to:
// VVV - Note the 'ref' keyword!
private static void RemoveXMLNode(ref XDocument doc, string descendant, string element, string elementValue)
You would then use the method like so:
// VVV - Note the 'ref' keyword!
RemoveXMLNode(ref doc, "Questions", "quPage", "PAGE60");
You can find more information on the difference between Pass By Reference and Pass By Copy over here.
There is also some more information on passing Reference Type classes from Jon Skeet's article over here.
I also wrote some dummy code to try out and demonstrate this behaviour over here.
2. Extension Method
This simply creates an extension method for your XDocument
object. You do so by telling the compiler which of your arguments' object you are extending using the this
keyword. When applying, you will actually reduce the number of required parameters by 1, effectively making it shorter.
The only requirement for this option is that your extension method(s) must be in a non-generic and non-nested static class.
You would write your method like so:
// VVVV - Note the 'this' keyword!
private static void RemoveXMLNode(this XDocument doc, string descendant, string element, string elementValue)
Now, you would call this method like so:
// Only three arguments!
doc.RemoveXMLNode("Questions", "quPage", "PAGE60");
$endgroup$
As a follow-up to t3chb0t's and your self-answer, I agree that whilst the original RemoveXMLNode
method you have written in your question is technically a one-liner, it is doing a lot.
In response to your self-answer, I have a few pointer:
- In your case, I don't think you need a separate
LoadXMLDocument
method. The lineXDocument.load(path)
is easy enough to understand. - Regarding you new
RemoveXMLNode
method, you have two options:
1. Pass by Reference
In your new method, you are requesting an XDocument
argument. Whilst XDocument
is a reference type and can be modified through its public methods, you may benefit from using the ref
keyword so that you pass the whole object as a reference. This means that any changes to the object you are passing happen on the original object. So to apply this, you would simply change the method arguments to:
// VVV - Note the 'ref' keyword!
private static void RemoveXMLNode(ref XDocument doc, string descendant, string element, string elementValue)
You would then use the method like so:
// VVV - Note the 'ref' keyword!
RemoveXMLNode(ref doc, "Questions", "quPage", "PAGE60");
You can find more information on the difference between Pass By Reference and Pass By Copy over here.
There is also some more information on passing Reference Type classes from Jon Skeet's article over here.
I also wrote some dummy code to try out and demonstrate this behaviour over here.
2. Extension Method
This simply creates an extension method for your XDocument
object. You do so by telling the compiler which of your arguments' object you are extending using the this
keyword. When applying, you will actually reduce the number of required parameters by 1, effectively making it shorter.
The only requirement for this option is that your extension method(s) must be in a non-generic and non-nested static class.
You would write your method like so:
// VVVV - Note the 'this' keyword!
private static void RemoveXMLNode(this XDocument doc, string descendant, string element, string elementValue)
Now, you would call this method like so:
// Only three arguments!
doc.RemoveXMLNode("Questions", "quPage", "PAGE60");
edited Feb 15 at 13:04
answered Feb 15 at 12:23
TomTom
1313
1313
4
$begingroup$
Regarding #1, you're wrong: becauseXDocument
is a reference type, the only thing that gets copied is a reference to theXDocument
instance, not the instance itself.
$endgroup$
– Pieter Witvoet
Feb 15 at 12:36
$begingroup$
@PieterWitvoet If you're referring to the fact that I mentioned that it's passing a copy, then (on further digging around) I agree and I will modify my answer. If you're referring to the use ofref
, I don't agree, as it still works.
$endgroup$
– Tom
Feb 15 at 12:57
1
$begingroup$
Addingref
won't break the code, but you'll be passing a reference to a reference around for no good reason. It'll clutter the code, send a confusing signal to other programmers and has a (tiny) performance implication. All for no benefit.
$endgroup$
– Pieter Witvoet
Feb 15 at 13:05
add a comment |
4
$begingroup$
Regarding #1, you're wrong: becauseXDocument
is a reference type, the only thing that gets copied is a reference to theXDocument
instance, not the instance itself.
$endgroup$
– Pieter Witvoet
Feb 15 at 12:36
$begingroup$
@PieterWitvoet If you're referring to the fact that I mentioned that it's passing a copy, then (on further digging around) I agree and I will modify my answer. If you're referring to the use ofref
, I don't agree, as it still works.
$endgroup$
– Tom
Feb 15 at 12:57
1
$begingroup$
Addingref
won't break the code, but you'll be passing a reference to a reference around for no good reason. It'll clutter the code, send a confusing signal to other programmers and has a (tiny) performance implication. All for no benefit.
$endgroup$
– Pieter Witvoet
Feb 15 at 13:05
4
4
$begingroup$
Regarding #1, you're wrong: because
XDocument
is a reference type, the only thing that gets copied is a reference to the XDocument
instance, not the instance itself.$endgroup$
– Pieter Witvoet
Feb 15 at 12:36
$begingroup$
Regarding #1, you're wrong: because
XDocument
is a reference type, the only thing that gets copied is a reference to the XDocument
instance, not the instance itself.$endgroup$
– Pieter Witvoet
Feb 15 at 12:36
$begingroup$
@PieterWitvoet If you're referring to the fact that I mentioned that it's passing a copy, then (on further digging around) I agree and I will modify my answer. If you're referring to the use of
ref
, I don't agree, as it still works.$endgroup$
– Tom
Feb 15 at 12:57
$begingroup$
@PieterWitvoet If you're referring to the fact that I mentioned that it's passing a copy, then (on further digging around) I agree and I will modify my answer. If you're referring to the use of
ref
, I don't agree, as it still works.$endgroup$
– Tom
Feb 15 at 12:57
1
1
$begingroup$
Adding
ref
won't break the code, but you'll be passing a reference to a reference around for no good reason. It'll clutter the code, send a confusing signal to other programmers and has a (tiny) performance implication. All for no benefit.$endgroup$
– Pieter Witvoet
Feb 15 at 13:05
$begingroup$
Adding
ref
won't break the code, but you'll be passing a reference to a reference around for no good reason. It'll clutter the code, send a confusing signal to other programmers and has a (tiny) performance implication. All for no benefit.$endgroup$
– Pieter Witvoet
Feb 15 at 13:05
add a comment |
$begingroup$
I went with the extension method in the end as it produced the least code
Extension method
public static class XDocumentExtensions
{
public static void RemoveXMLNode(this XDocument doc, string descendant, string element, string elementValue)
{
doc.Descendants(descendant)
.Where(n => (string)n.Element(element) == elementValue)
.Remove();
}
}
Main program
class Program
{
static void Main(string args)
{
XDocument doc = XDocument.Load(@"C:TempOriginal.xml");
doc.RemoveXMLNode("Questions", "quPage", "PAGE60");
doc.Save(@"C:TempNewXmlDoc.xml");
}
}
$endgroup$
$begingroup$
I would add theref
keyword to theXDocument
argument in theRemoveXMLNode
method, so that you actually pass by reference. As it is, it will create a copy ofdoc
and remove the node from the copy. The original will stay with the node you are trying to remove.
$endgroup$
– Tom
Feb 15 at 11:51
5
$begingroup$
@Tom:XDocument
is a class and thus a reference type. What you are saying only applies to value types.
$endgroup$
– Pieter Witvoet
Feb 15 at 12:24
$begingroup$
While OPs are encouraged to answer their own questions bear in mind that "Every answer must make at least one insightful observation about the code in the question. Answers that merely provide an alternate solution with no explanation or justification do not constitute valid Code Review answers and may be deleted."...
$endgroup$
– Sᴀᴍ Onᴇᴌᴀ
Feb 15 at 18:10
add a comment |
$begingroup$
I went with the extension method in the end as it produced the least code
Extension method
public static class XDocumentExtensions
{
public static void RemoveXMLNode(this XDocument doc, string descendant, string element, string elementValue)
{
doc.Descendants(descendant)
.Where(n => (string)n.Element(element) == elementValue)
.Remove();
}
}
Main program
class Program
{
static void Main(string args)
{
XDocument doc = XDocument.Load(@"C:TempOriginal.xml");
doc.RemoveXMLNode("Questions", "quPage", "PAGE60");
doc.Save(@"C:TempNewXmlDoc.xml");
}
}
$endgroup$
$begingroup$
I would add theref
keyword to theXDocument
argument in theRemoveXMLNode
method, so that you actually pass by reference. As it is, it will create a copy ofdoc
and remove the node from the copy. The original will stay with the node you are trying to remove.
$endgroup$
– Tom
Feb 15 at 11:51
5
$begingroup$
@Tom:XDocument
is a class and thus a reference type. What you are saying only applies to value types.
$endgroup$
– Pieter Witvoet
Feb 15 at 12:24
$begingroup$
While OPs are encouraged to answer their own questions bear in mind that "Every answer must make at least one insightful observation about the code in the question. Answers that merely provide an alternate solution with no explanation or justification do not constitute valid Code Review answers and may be deleted."...
$endgroup$
– Sᴀᴍ Onᴇᴌᴀ
Feb 15 at 18:10
add a comment |
$begingroup$
I went with the extension method in the end as it produced the least code
Extension method
public static class XDocumentExtensions
{
public static void RemoveXMLNode(this XDocument doc, string descendant, string element, string elementValue)
{
doc.Descendants(descendant)
.Where(n => (string)n.Element(element) == elementValue)
.Remove();
}
}
Main program
class Program
{
static void Main(string args)
{
XDocument doc = XDocument.Load(@"C:TempOriginal.xml");
doc.RemoveXMLNode("Questions", "quPage", "PAGE60");
doc.Save(@"C:TempNewXmlDoc.xml");
}
}
$endgroup$
I went with the extension method in the end as it produced the least code
Extension method
public static class XDocumentExtensions
{
public static void RemoveXMLNode(this XDocument doc, string descendant, string element, string elementValue)
{
doc.Descendants(descendant)
.Where(n => (string)n.Element(element) == elementValue)
.Remove();
}
}
Main program
class Program
{
static void Main(string args)
{
XDocument doc = XDocument.Load(@"C:TempOriginal.xml");
doc.RemoveXMLNode("Questions", "quPage", "PAGE60");
doc.Save(@"C:TempNewXmlDoc.xml");
}
}
edited Feb 15 at 17:09
community wiki
Syntax Error
$begingroup$
I would add theref
keyword to theXDocument
argument in theRemoveXMLNode
method, so that you actually pass by reference. As it is, it will create a copy ofdoc
and remove the node from the copy. The original will stay with the node you are trying to remove.
$endgroup$
– Tom
Feb 15 at 11:51
5
$begingroup$
@Tom:XDocument
is a class and thus a reference type. What you are saying only applies to value types.
$endgroup$
– Pieter Witvoet
Feb 15 at 12:24
$begingroup$
While OPs are encouraged to answer their own questions bear in mind that "Every answer must make at least one insightful observation about the code in the question. Answers that merely provide an alternate solution with no explanation or justification do not constitute valid Code Review answers and may be deleted."...
$endgroup$
– Sᴀᴍ Onᴇᴌᴀ
Feb 15 at 18:10
add a comment |
$begingroup$
I would add theref
keyword to theXDocument
argument in theRemoveXMLNode
method, so that you actually pass by reference. As it is, it will create a copy ofdoc
and remove the node from the copy. The original will stay with the node you are trying to remove.
$endgroup$
– Tom
Feb 15 at 11:51
5
$begingroup$
@Tom:XDocument
is a class and thus a reference type. What you are saying only applies to value types.
$endgroup$
– Pieter Witvoet
Feb 15 at 12:24
$begingroup$
While OPs are encouraged to answer their own questions bear in mind that "Every answer must make at least one insightful observation about the code in the question. Answers that merely provide an alternate solution with no explanation or justification do not constitute valid Code Review answers and may be deleted."...
$endgroup$
– Sᴀᴍ Onᴇᴌᴀ
Feb 15 at 18:10
$begingroup$
I would add the
ref
keyword to the XDocument
argument in the RemoveXMLNode
method, so that you actually pass by reference. As it is, it will create a copy of doc
and remove the node from the copy. The original will stay with the node you are trying to remove.$endgroup$
– Tom
Feb 15 at 11:51
$begingroup$
I would add the
ref
keyword to the XDocument
argument in the RemoveXMLNode
method, so that you actually pass by reference. As it is, it will create a copy of doc
and remove the node from the copy. The original will stay with the node you are trying to remove.$endgroup$
– Tom
Feb 15 at 11:51
5
5
$begingroup$
@Tom:
XDocument
is a class and thus a reference type. What you are saying only applies to value types.$endgroup$
– Pieter Witvoet
Feb 15 at 12:24
$begingroup$
@Tom:
XDocument
is a class and thus a reference type. What you are saying only applies to value types.$endgroup$
– Pieter Witvoet
Feb 15 at 12:24
$begingroup$
While OPs are encouraged to answer their own questions bear in mind that "Every answer must make at least one insightful observation about the code in the question. Answers that merely provide an alternate solution with no explanation or justification do not constitute valid Code Review answers and may be deleted."...
$endgroup$
– Sᴀᴍ Onᴇᴌᴀ
Feb 15 at 18:10
$begingroup$
While OPs are encouraged to answer their own questions bear in mind that "Every answer must make at least one insightful observation about the code in the question. Answers that merely provide an alternate solution with no explanation or justification do not constitute valid Code Review answers and may be deleted."...
$endgroup$
– Sᴀᴍ Onᴇᴌᴀ
Feb 15 at 18:10
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f213509%2fxml-node-removal-method-with-5-arguments%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
W,OjPAKGmrqsBbTRZqBl8CpCpMSrQLQr woGHedTg2ji5DJHU75t4X Ap5meo